Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial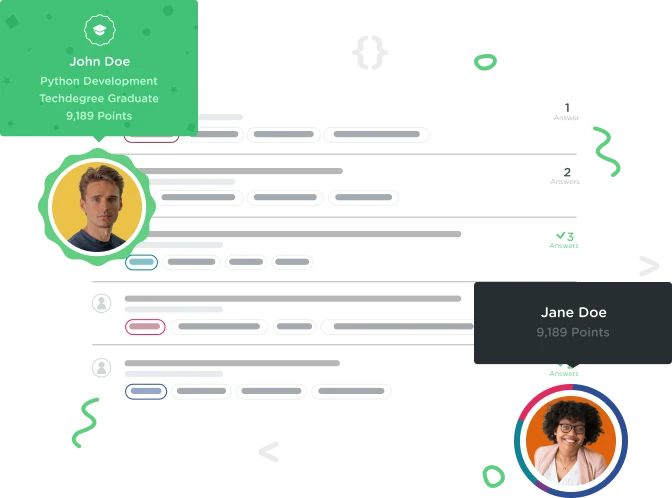
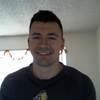
Silviu Popovici
20,433 PointsWhat do you guys think of my solution?
Went a bit wild on this one. I added the ability for the user to search for a name as well as the ability to type "ALL" and display all the users' data like in the last challenge. What do you guys think of my solution?
var students = [
{
name: "Silv",
track: "Javascript",
achievements: 39,
points: 7500
},
{
name: "Bob",
track: "Front-end",
achievements: 56,
points: 2300
},
{
name: "Mary",
track: "Ruby",
achievements: 66,
points: 8500
},
{
name: "Josh",
track: "Rails",
achievements: 58,
points: 9500
},
{
name: "Joe",
track: "Java",
achievements: 16,
points: 1500
}
];
//Lists all student data
function fullStudentListing()
{
for(var i=0; i < students.length; i++){
for(prop in students[i])
{
document.write(prop + ": " + students[i][prop] + "<br>");
}
document.write("<br>");
}
}
//Lists data for one single student
function singleStudentListing(num){
var temp = "";
for (prop in students[num]){
temp += prop + ": " + students[num][prop] + "<br>";
}
temp += "<br>"
return temp;
}
//Searches student data for given name and
//returns either a listing or a "not found" error
function linearSearch(searchTerm){
for(var i=0; i < students.length; i++)
{
if(students[i].name.toUpperCase() === searchTerm.toUpperCase()){
return singleStudentListing(i);
}
}
return "Error: Name " + searchTerm + " not found <br>";
}
//Main Loop
do{
var name = prompt("Enter a student's name to see their data, enter ALL to list all students, or enter QUIT to exit");
if(name.toUpperCase() !== "QUIT" && name.toUpperCase() !== "ALL"){
document.write(linearSearch(name));
}
else if(name.toUpperCase() == "ALL")
{
fullStudentListing();
}
} while(name.toUpperCase() !== "QUIT")
2 Answers
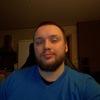
Meelis Talvis
2,007 PointsFunctions look good! You could only play around and change the main loop but that won't improve the efficiency of the code much. Good work!

Piotr Połaniecki
Courses Plus Student 3,181 PointsThanks Silviu,
Your code helped me a lot to understand step by step what is really going on. Ability to write short snippets is one thing, writing more complex program is something completely different. Much harder for me. Thanks!