Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial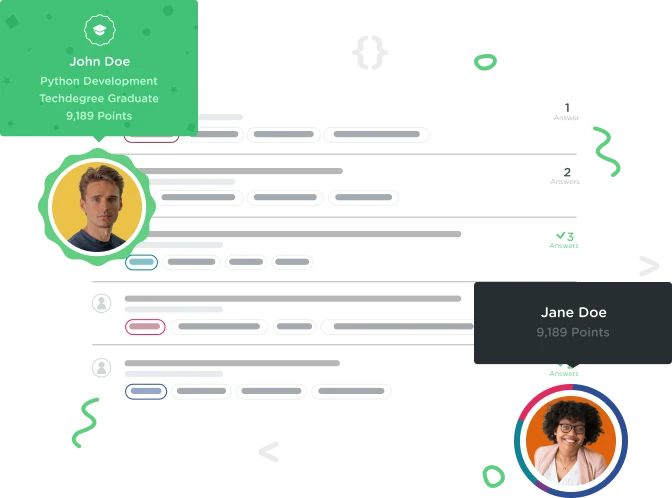
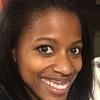
nicole lumpkin
Courses Plus Student 5,328 PointsWhat do you think about my code?
I really struggled with this one and used previous Community posts to see some example code. I was able to work my way through another student's code, then took a day off, then this is what I came up with without referring back to other posts. My code passed, but I'm concerned my solution is weird lol. Would anyone weigh in!?
class Hand(list):
def __init__(self, num=0, die_class = D20):
self.num = num
super().__init__()
for _ in range(num):
self.append(die_class())
@property
def total(self):
return sum(self)
@classmethod
def roll(cls, num):
return cls(num)
2 Answers
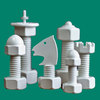
Steven Parker
230,274 PointsRemember, the instructions said, "I don't care how you do it, I only care that it works."
I've seen many questions on this specific challenge, and all sorts of different solutions. But generally there's two categories of solution approaches: override __init__
and have a minimal roll
(such as what you did), or put all the new code into roll
. Correctly done, both pass the challenge, and are equally good solutions.
But if you wanted a little extra practice, you could try solving it the other way.
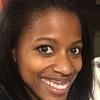
nicole lumpkin
Courses Plus Student 5,328 PointsHere's my alternate solution(which passed)!! Thanks for challenging me to try!
from dice import D20
class Hand(list):
def __init__(self, res):
super().__init__()
self.extend(res)
@property
def total(self):
return sum(self)
@classmethod
def roll(cls, num, die_class = D20):
res = []
for _ in range(num):
res.append(die_class())
return cls(res)
QUESTION: I wrote another version, one in which if a Hand object were created outside of a class method...
hand = Hand()
...the instantiation of said Hand object would NOT require a res argument(unlike the code above). It seems to work in my IDE but is failing to pass. The reason given was 'Didn't get correct value for hand.total'. Would anyone mind playing around with the code below to see why this is happening!?
class Hand(list):
def __init__(self, res = None):
super().__init__()
try:
self.extend(res)
except TypeError:
self = []
@property
def total(self):
return sum(self)
@classmethod
def roll(cls, num, die_class = D20):
res = []
for _ in range(num):
res.append(die_class())
return cls(res)
Here is me playing around with it and it seems to work.
hand = Hand()
hand.total
Out[198]: 0
hand.extend([1,2,3])
hand.total
Out[200]: 6
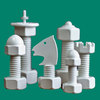
Steven Parker
230,274 PointsYou've certainly gotten a lot of good extra practice from this challenge!
nicole lumpkin
Courses Plus Student 5,328 Pointsnicole lumpkin
Courses Plus Student 5,328 PointsThank you Steven, that was just what I was trying to ask!!