Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial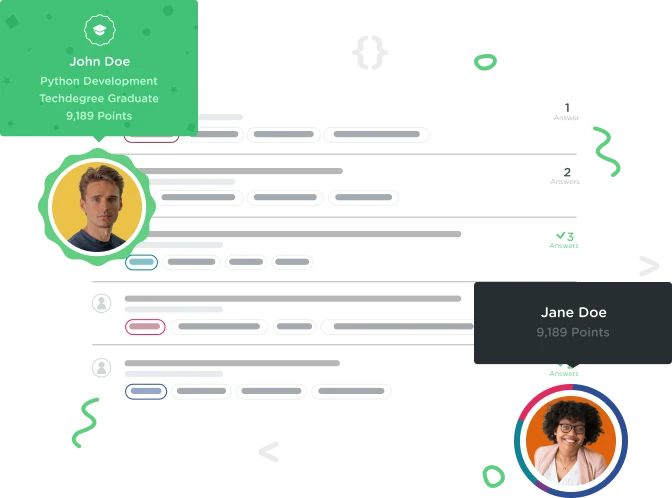
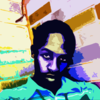
MUZ141095 Kelvin Chawora
9,348 PointsWhat do you think about my Python in the Dungeon game...???
Hello Pythonistas
So i'm doing python, and i finished Python Collections, and created my own dungeon game.. Its kinda boring that i don't have anyone to show it off to.. So, here we go.
import random
#room grid
ROOM_GRID = [(1,1), (1,2), (1,3), (1,4), (1,5),
(2,1), (2,2), (2,3), (2,4), (2,5),
(3,1), (3,2), (3,3), (3,4), (3,5),
(4,1), (4,2), (4,3), (4,4), (4,5),
(5,1), (5,2), (5,3), (5,4), (5,5)]
# Keep track of where the player has been
player_trail = []
#creating get_locations
def get_locations():
start_location = random.choice(ROOM_GRID)
monster_location = random.choice(ROOM_GRID)
door_location = random.choice(ROOM_GRID)
if monster_location == door_location or monster_location == start_location or door_location == start_location:
return get_locations()
return start_location, monster_location, door_location
#function that prints the map
def print_map():
count = 0
printed_map = []
while count < len(ROOM_GRID):
current_room = ROOM_GRID[count]
if current_room == player_location:
printed_map.append('O')
elif current_room in player_trail:
printed_map.append('.')
else:
printed_map.append('~')
if current_room[1] == 5:
printed_map.append("\n")
count += 1
print(''.join(printed_map))
# Get the locations
player_location, monster_location, door_location = get_locations()
player_trail.append(player_location)
#scary greeting
print("Welcome to the Dungeon! Muaaaaaaahhahahahahahahahahahahahaaaaaa")
print("Direction keys: 'a' for left, 'd' for right, 'x' for down and 'w' for up")
print("Just pretend/visualise W-A-S-D as the d-pad for controls...")
print("----------------------------------------------------")
print("----------------------------------------------------")
#calling the function to print the map after the greeting
print_map()
while True:
move = input("Which direction do you wanna go...? ")
print("----------------------------------------------------")
move = move.lower()
# Move left
if move == "a" and player_location[1] > 1:
player_location = player_location[0], player_location[1]-1
# Move right
elif move == "d" and player_location[1] < 5:
player_location = player_location[0], player_location[1]+1
# Move down
elif move == "s" and player_location[0] < 5:
player_location = player_location[0]+1, player_location[1]
# Move up
elif move == "w" and player_location[0] > 1:
player_location = player_location[0]-1, player_location[1]
else:
print("Please follow instructions to control the player")
print("----------------------------------------------------")
print("Direction keys: 'a' for left, 'd' for right, 'x' for down and 'w' for up")
player_trail.append(player_location)
if player_location == monster_location:
print_map()
print("Oh snap! The Python got you!")
print("-------GAME OVER--------")
print(" :( ")
break
if player_location == door_location:
print_map()
print("Yay! You escaped the silly python....!")
print("*********************")
print("******YOU WIN********")
print("*********************")
break
print_map()
Feel free to review it and pass comments...