Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial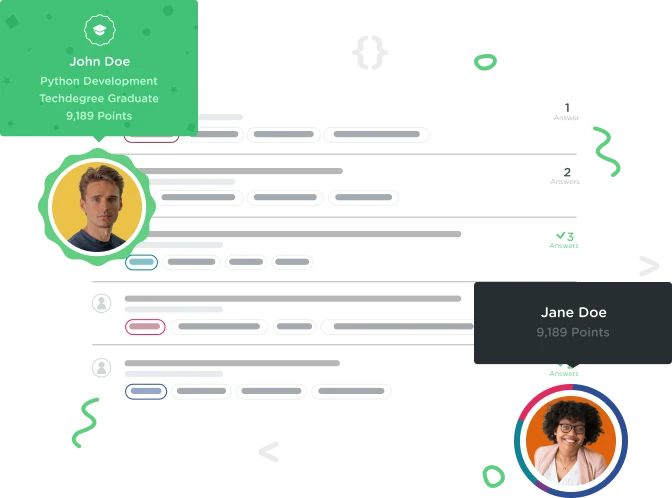

Udo Wasserfuhr
2,614 PointsWhat do you think of my solution?
Hey there,
here's my solution:
def vowelmonster(vowel_list, input_list):
for word in input_list:
wordlist = list(word) #split each word into a list of chars
i = 0
while i < len(vowel_list): #for each char in vowel_list, try to remove this char
#one by one until an exception is thrown
try:
wordlist.remove(vowel_list[i])
except ValueError:
i += 1
wordlist[0] = wordlist[0].upper() #capitalize first letter
new_word = "".join(wordlist)
output_list.append(new_word)
return output_list
input_list = ["hello", "it", "is", "me", "wondering", "the", "things", "treehouse"]
vowel_list = ["a", "e", "i", "o", "u"]
output_list = []
print("Here\'s your list without vowels:")
print(vowelmonster(vowel_list, input_list))
What do you think? :)
1 Answer
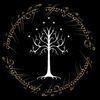
jacinator
11,936 PointsI made a few upgrades. A few things to not are the conventions on blank lines. Usually cap it at one inside of a function. It looks better. Also removed the while loop and the try/except. Also you didn't need to pass in vowel_list
or even have it as a list. You also don't need to define output_list
outside of vowelmonster
.
Your use of join
and for
loops is well done. I realize that I'm not using join
as you are, but it's a good tool that you're drawing on.
def vowelmonster(input_list):
output_list = []
for word in input_list: # Loop over the list of words
new_word = ""
for char in word.lower(): # Loop over the characters in the word
if char not in "aeiou": # If the character isn't a vowel...
new_word += char # ...Add the character to the new word
output_list.append(new_word.capitalize()) # Add the new word to the output list
return output_list
input_list = ["hello", "it", "is", "me", "wondering", "the", "things", "treehouse"]
print("Here's your list without vowels:")
print(vowelmonster(input_list))
You're code is pretty well thought out and functional. As you get more practice you'll learn more of Python's builtin tools. Like your capitalization trick as opposed to what I used. These are just things that you'll learn over time. One thing that I encourage you towards is beautiful code. If it doesn't look good, chances are it isn't the best way.
The last thing I would say, beautiful code is good code. You'll thank yourself if no-one else does.
fahad lashari
7,693 Pointsfahad lashari
7,693 PointsWow very clever. Good use of the try and except. I wish I had thought of that. I couldn't think of a way of solving this with try and except.