Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial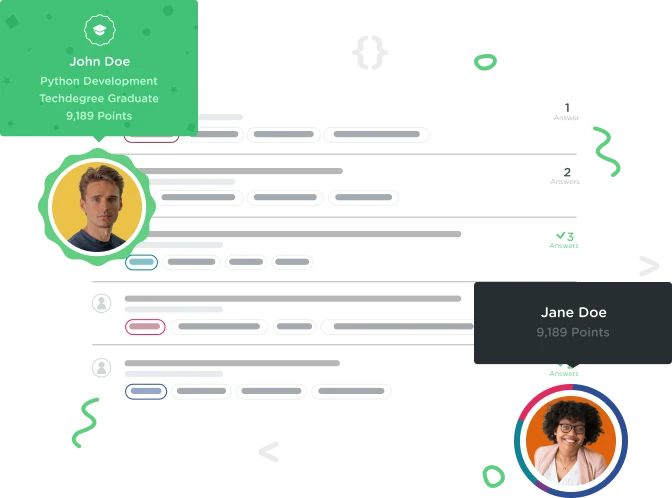

erdragerdragsson
Courses Plus Student 5,887 PointsWhat do you think of this?
what do you think of this? (and check out my question at the bottom :) )
var score = 0;
var firstQuestion = prompt("Whats the capital city of sweden?");
if (firstQuestion.toLowerCase() === "stockholm") {
score += 1;
alert("Correct, you have earned 1 point");
} else {
alert("Wrong! the correct answer is stockholm");
}
var secondQuestion = prompt("Whats the capital city of Norway?");
if (secondQuestion.toLowerCase() === "oslo") {
score += 1;
alert("Correct, you have earned 1 point");
} else {
alert("Wrong! the correct answer is oslo");
}
var thirdQuestion = prompt("Whats the capital city of Denmark?");
if (thirdQuestion.toLowerCase() === "kopenhagen") {
score += 1;
alert("Correct, you have earned 1 point");
} else {
alert("Wrong! the correct answer is kopenhagen");
}
var fourthQuestion = prompt("Whats the capital city of Finland?");
if (fourthQuestion.toLowerCase() === "helsinki") {
score += 1;
alert("Correct, you have earned 1 point");
} else {
alert("Wrong! the correct answer is helsinki");
}
var fifthQuestion = prompt("Whats the capital city of England?");
if (fifthQuestion.toLowerCase() === "london") {
score += 1;
alert("Correct, you have earned 1 point");
} else {
alert("Wrong! the correct answer is london");
}
if (score === 5) {
alert(" Congratulaions you earned a golden crown!");
} else if (score === 4 || score === 3) {
alert("Good job your earned a Silver crown!");
} else if (score === 2 || score === 1) {
alert("Good, you earned a bronze crown!");
} else {
document.write("You did not answer any questions right, you did not earn a crown!");
}
if(alert) {
alert("Refresh the page to try again")
document.write('<A HREF="javascript:location.reload(true)">Reload the page!</A>')
}
one thing i wonder doe, is when i first did this i put all the question variables at the top together, like this
var firstQuestion = prompt("Whats the capital city of sweden?");
var secondQuestion = prompt("Whats the capital city of Norway?");
var thirdQuestion = prompt("Whats the capital city of Denmark?");
var fourthQuestion = prompt("Whats the capital city of Finland?");
var fifthQuestion = prompt("Whats the capital city of England?");
but when i did that, the whole code, ran wierd, and i wonder why it does that, try it out yourself, remove all the question variables over the "if" statements and add them at the top,

erdragerdragsson
Courses Plus Student 5,887 Pointsso if i have a variable with a function like prompt, i shouldn't but them at the top? as they would run before everything else?
and also, what did you think of the program for the video challenge? :)
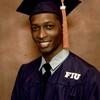
Dane Parchment
Treehouse Moderator 11,075 PointsWhen it comes to anything that outputs (alerts, prompts, etc.) place them when you want them to appear, so if you want them to appear at the very beginning of script before any checks are made place them in the beginning, if you want them to appear after a user clicks a button place them in the function of the button click .....you get the point.
Pretty Good!

erdragerdragsson
Courses Plus Student 5,887 Pointsokey thanks for explaining it!:)
i appreciate it
// erdrag
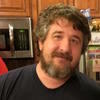
Michael Hall
Courses Plus Student 30,909 PointsThank you for this! I was trying to figure out what was going on. I had all my variables at the top. Thanks for the explanation Dane, makes total sense.
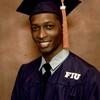
Dane Parchment
Treehouse Moderator 11,075 PointsNo problem glad to be of some help. :D
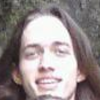
Andrew Gay
20,893 PointsPost your comments (That are meant to be answers) as an answer so a best answer can be selected.
1 Answer
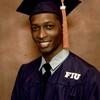
Dane Parchment
Treehouse Moderator 11,075 PointsWell you have to remember that javascript runs its code from top to bottom. So when you put all of your variables at the top javascript will initialize them. Because you gave the variables a value the variables are initialized before the rest of the code can be read. Generally this isn't a problem as you should generally place your variables at the top of all your scripts/functions. However, in your case you set your variables equal to a function, so when the code ran over your variables it initialized each one ran its function (which opens the prompt screen). Unfortunately because the rest of your code is read after each of your variables are intitialized it wouldn't do anything other than open up a few prompts.
Dane Parchment
Treehouse Moderator 11,075 PointsDane Parchment
Treehouse Moderator 11,075 PointsWell you have to remember that javascript runs its code from top to bottom. So when you put all of your variables at the top javascript will initialize them. Because you gave the variables a value the variables are initialized before the rest of the code can be read. Generally this isn't a problem as you should generally place your variables at the top of all your scripts/functions. However, in your case you set your variables equal to a function, so when the code ran over your variables it initialized each one ran its function (which opens the prompt screen). Unfortunately because the rest of your code is read after each of your variables are intitialized it wouldn't do anything other than open up a few prompts.