Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial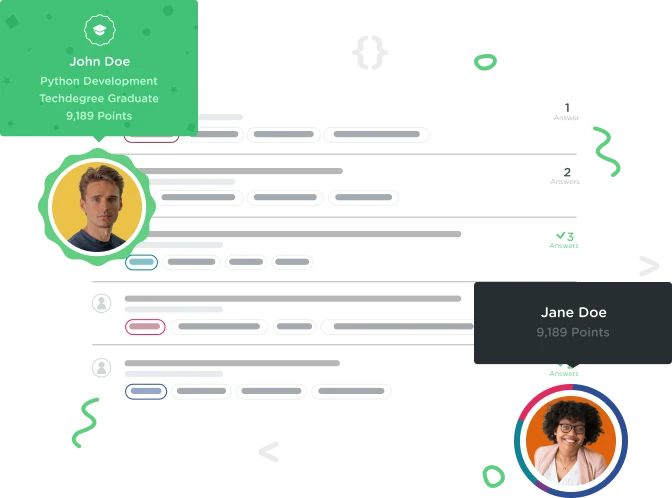

alexandraz
9,587 PointsWhat do you think of this slightly different solution?
I did a list variable called Strawman with '_' characters in it and every time a guess is correct I assign it to the list with the index of the correct letter in the secret word. Does that make sense? It might be a bit more complicated than the solution in the video but I think it's nice that the for loop runs only when the guess is a hit.
import random
# make a list of words
words = [
'apple',
'banana',
'orange',
'coconut',
'lemon',
'blueberry',
'melon'
]
while True:
start = input("Press enter to start or enter Q to quit ")
if start.lower() == 'q':
break
# pick a random word
secret_word = random.choice(words)
bad_guesses = []
good_guesses = []
# draw spaces
strawman = list("_"*len(secret_word))
print("".join(strawman))
while len(bad_guesses) < 7 and len(secret_word) > len(good_guesses):
# take a guess
guess = input("Guess a letter: ")
if guess.isalpha() is False:
print("Please type alphabetic characters only")
elif guess in good_guesses or guess in bad_guesses:
print("You've already guessed that letter!")
# draw guessed letters and strikes
elif guess in secret_word:
for letter in secret_word:
if letter == guess:
strawman[secret_word.index(letter)] = guess
good_guesses.append(guess)
else:
bad_guesses.append(guess)
print("".join(strawman))
print("Strikes: {}/7".format(len(bad_guesses)))
# print out win/lose
if len(bad_guesses) == 7:
print("You lost!")
else:
print("You won! the correct word was: " + secret_word)