Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial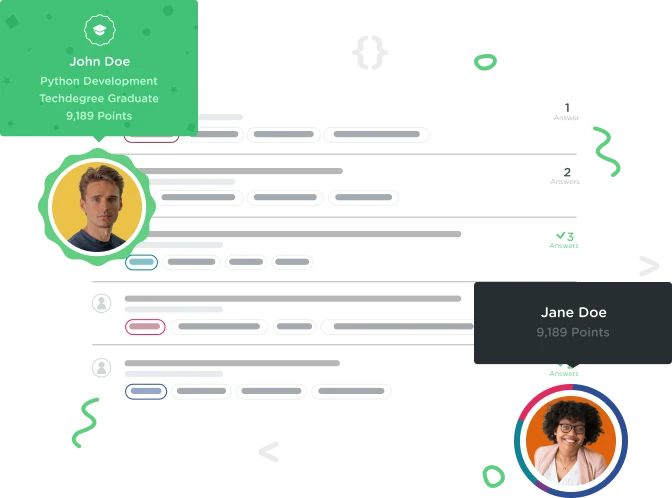
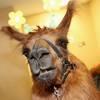
A X
12,842 PointsWhat does a Return do?
I just finished watching Jason's Method Returns video, and for me he didn't adequately explain what a return keyword does. He only mentions that a return: "(when calling a method) we have the option to get data or variables back from the method."
In the following sample code from that video you'll see that only the first call of the method that has puts add
returns anything...so what happens to the values of the add (5,5) and add (6,3)? Are they stored? Are they available in the console in a web browser to see (like in Chrome console)? Does nothing happen? Something else?
def add (a,b)
puts "Adding #{a} and #{b}:"
return a + b
end
puts add (2, 3)
add (5, 5)
add (6,3)
Thanks for your help!
1 Answer
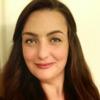
Jennifer Nordell
Treehouse TeacherÏn the code you've written here ... no, they're not stored. But they could be! Take this line for example:
result = add(3, 20)
We would take 3 and 20 and send them away to our add function. Then when the add function runs with those two numbers it runs any code in there. That code could be just about anything, but in this scenario it simply adds the two numbers together. So you have the code return a + b
. This will return the value of a + b (23 in this scenario) to the thing that first called it. Now result will be equal to 23.
Keep in mind that some programming languages allow you to return multiple items and some do not. As I understand it ruby does not support returning more than one item at a time. However, that item could be a list of other items (like an array). Hope that helps!
A X
12,842 PointsA X
12,842 PointsI didn't realize that Ruby (potentially) doesn't support returning more than 1 item at a time...that seems odd that they would create that restriction...but good information to know moving forward! Thanks for your response!
A X
12,842 PointsA X
12,842 PointsAlso a follow up question to your example, so we get the result of 23, is that value (23) now stored in the
def add
as part of what the return statement does? Or is the Ruby code run and then the value of 23 "forgotten"?Jennifer Nordell
Treehouse TeacherJennifer Nordell
Treehouse TeacherOnce the function runs and exits, that information is gone! So if you want your return to mean anything it either has to be stored outside the function or do some sort of print or something in the function which would display to the user. This is what you'll see also in other languages.
Keep in mind also that returning multiple items from a return statement is fairly new in programming. There are still many languages that can only return one single thing. The two exceptions I know to this rule right now are Python and Swift. There may be more, of course.
Tanja Stroble
Courses Plus Student 1,003 PointsTanja Stroble
Courses Plus Student 1,003 PointsFrom your example above, is result now a variable whose value is the result of add method ? If so, is it reusable and can it be interpolated into a string? Thanks