Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial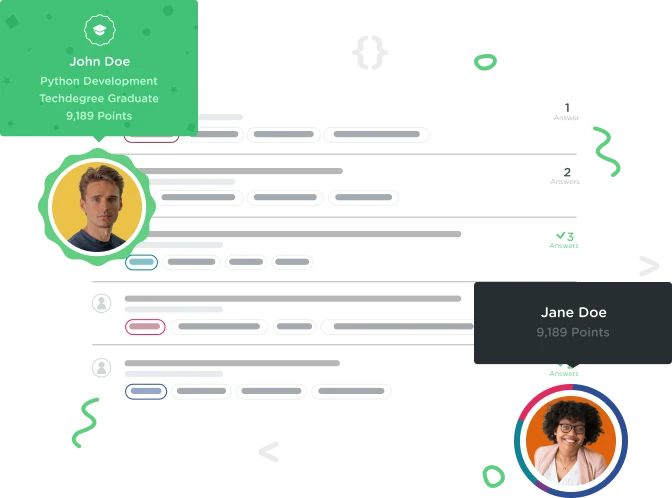
Drew Carver
5,189 PointsWhat does he mean by "byte representation" of the sound?
I've been trying to understand what exactly the SystemSoundID
is in
var sound: SystemSoundID = 0
He says it's a byte representation of the sound file. Does this mean the actual location in memory of where the sound file is? In other words, if
AudioServicesCreateSystemSoundID(soundURL, &sound)
makes
sound = 4038
is 4038 the memory address of the wav file?
I've watched this video multiple times to try and understand this, and there's something I'm not getting on it.
1 Answer

Sahil Gangele
6,851 PointsIn your case 4038 will be the byte representation of the .wav file.
SystemSoundID
variable contains the actual byte representation of the .wav file.
The key is realizing that SystemSoundID
is UInt32
. This means that it is a value type and not a reference type.
In the function AudioServicesCreateSystemSoundID(soundURL, &sound)
:
-
soundURL
: .wav File which is represented in memory as a sequence of bytes. eg. 00110101 00111100 etc. -
sound
: The actual value of the sound variable (The bytes) -
&sound
: The current address of the sound variable
And an address is simply the location of this variable on the computer (Technically Stack/Heap, but computer will suffice). This variable has to be stored somewhere right!? And how does the computer know where to look for a variable? It uses addresses.
Why do we need &sound
? Well... The function is trying to copy over the byte representation of the .wav file into the sound
variable. How will it do this!? Parameters in Swift are local copies, and since our sound variable is a value type of UInt32
, how will that function copy over the byte representation of the .wav file to the sound variable in the function? If it tries to do this:
Class SoundEffectsPlayer {
var sound: SystemSoundID = 0
func playSound(for story: Story) {
let soundURL = story.soundEffectURL as CFURL
AudioServicesCreateSystemSoundID(soundURL, sound)
}
}
// Some what pseudocode
AudioServicesCreateSystemSoundID( _ file: .wav, _ sound: SystemSoundID) {
copyBytes(from: .wav, to: sound)
}
// Once you leave the function, the sound variable will not be changed!
// It will still have its original value of 0!
// This is because sound is a local variable & it's a copied value. It's not the original sound variable being used.
So how do we fix this? Addresses!
By giving the address of the sound variable, we let the function make changes to the sound variable and have those changes be kept after the function closes. That's because it's not accessing your local paramter sound... no. It's accessing the address... which lets it know where the variable is on the computer (Stack/Heap).
Ultimately It allows the function to make changes to the original sound variable anywhere, and make changes to the value of the sound variable.
Drew Carver
5,189 PointsDrew Carver
5,189 PointsWhat's interesting though is if I make the function print the SystemSoundID (the variable
sound
) to the console, the number goes up sequentially every time the function is called (every time the button is pressed in the app). It looks like:4097 4098 4099 4100 4101 4102 4103
If it's a byte representation of the .wav file, why would it change every time the same exact file is called? It almost seems like the SystemSoundID is an arbitrary ID being assigned to the sound file, rather than any kind of byte representation of its actual data.