Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial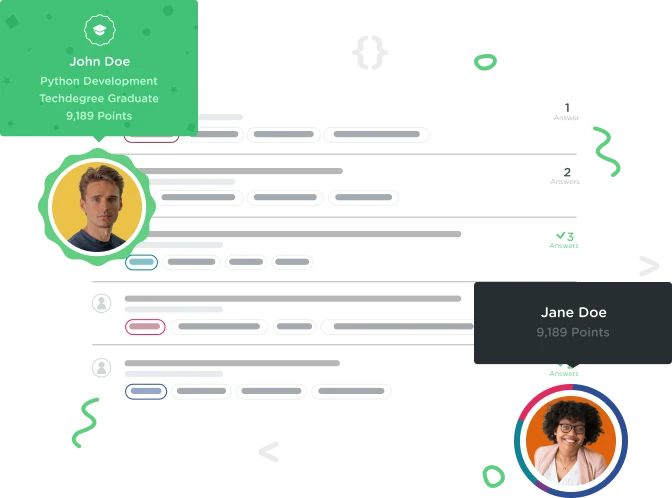

Roland Shen
7,628 PointsWhat does "If let result = (some function here)" do?
import UIKit
func isDivisible(numberOne: Int, numberTwo: Int) -> Bool? { if numberOne % numberTwo == 0 { return true } else { return nil
}
}
if let result = isDivisible(4, 2) { println("Divisible") } else { println("Not Divisible") }
So here's the code that was the correct answer for this exercise. I'm confused on what this line does: if let result = isDivisible(4, 2) { Does this call the function isDivisible with the parameters and store it in result? If so, then how come it will print Not Divisible when the numbers in the parameter don't divide? What part in the isDivisible function occurs to cause the Not Divisible to be printed? Does the nil thing make it goto the else in the second if else?
2 Answers

Di Su
Courses Plus Student 1,068 PointsHi Roland,
if let result = isDivisible(4, 2) is used to check if the optional does not equal nil.
If the optional does not equal nil, then it must equal true, therefore println("Divisible")
If the optional does not equal true, then it must equal nil, therefore println("Not Divisible")
So for example, we could do:
if isDivisible(firstNumber: 4, secondNumber: 2) != nil {
println("Divisible")
} else {
println("Not Divisible")
}
where != translates to 'does not equal'.
This works because our goal is to check to see if a value has been returned from our function. The actual value does not matter.
So for example, we could do:
func isDivisible(#firstNumber: Int, #secondNumber: Int) -> Int? {
if firstNumber % secondNumber == 0 {
return 5
}
return nil
}
if isDivisible(firstNumber: 4, secondNumber: 2) != nil {
println("Divisible")
} else {
println("Not Divisible")
}
This will println("Divisible") because our function has returned a value and does not equal nil.
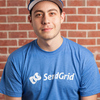
Dylan Shine
17,565 PointsSwift uses a thing called Optionals, which in laymen's terms allow Swift to gracefully handle an absence of a value (aka nil). When you write an if let statement, you are checking to make sure that your value isn't nil.
Example:
let favFood = ["Pizza", "Ice Cream"]
if let result = favFood[1] {
println("Yum")
} // this will execute
if let result = favFood[2] {
println("Yum")
} //This will not execute, favFood[2] doesn't exist

Roland Shen
7,628 PointsI see, but how is that example using an optional? Imgur in this code written here, how can the 'return true' from the isDivisible function translate to "Divisible" and how does nil translate to "Not Divisible"? I think I have some of it figured out with the optionals stuff, but what does result mean then?
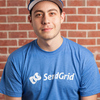
Dylan Shine
17,565 PointsHi Ronald,
When you add a "?" to the end of your functions Bool return type, you are saying that it's okay for the function to return a Bool (true or false) or nil. If you were to remove the question mark, it would through an error. Think of it like an optional value, Int? is an integer with the option to be nil or String? a string with the option to be nil.