Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial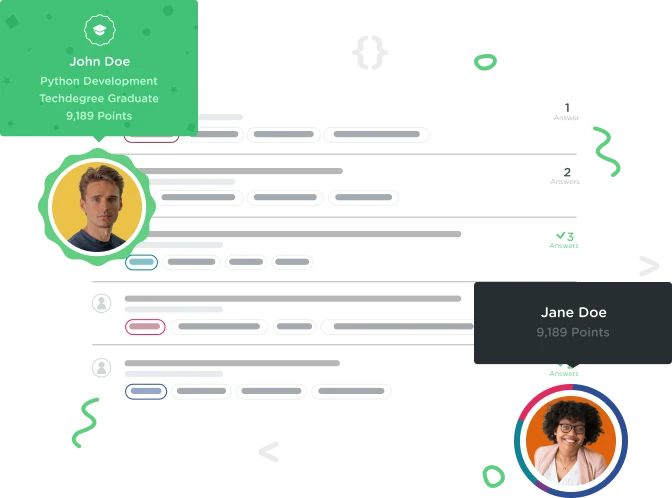
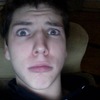
Charlie Person
8,692 PointsWhat does Jim mean at 9:34 when he says "but remember functions are objects"?
I don't get it. Can someone please explain how exactly functions are objects??? I understand that methods are functions that are properties of an object. But now he's saying that functions are objects and it's really throwing me for a bit of a loop. Would really appreciate some help.
3 Answers
William Li
Courses Plus Student 26,868 PointsYeah, in functional programming, you'll often hear about the term higher order function, meaning, function was treated as first class citizen; that's true too in JavaScript, function is THE first-class object, here is why.
1 because function really is object
function addTwo(a, b) {
return a + b;
}
addTwo instanceof Object
// => true
2 Like object, a function, once defined, has its own properties and methods through prototype chain from Function.prototype.
addTwo.name;
// => addTwo
addTwo.toString();
// => "function addTwo(a, b) {return a + b;}"
Do these two lines look familiar to you? They should be, since they are how you access an object's property and method using dot notation.
3 Functions, like numbers, array, or any other objects in JavaScript, can be assigned to a variable or pass in as argument to another function.
var f = addTwo; // assign function to variable f
function mulTwo(a,b) {return a * b;}
function applyFunc(a, b, func) { // pass in function as argument.
return func(a,b);
}
applyFunc(3,4, addTwo); // => 7
applyFunc(3,4, mulTwo); // => 12
So there you have it, function in JavaScript really is just object, it has all the object's characteristics, and it can do what any object can. When programming in JavaScript, the sooner you begin to think and treat function as object, the better off you will be, because this concept of function as first-class object is used alot when writing JavaScript code.

Andrew Barrette
Courses Plus Student 2,219 PointsWilliam's answer is pretty thorough, but here is something that really helped me with this concept. For me, it was particularly helpful in understanding functions as objects when I learned that the following two lines of code are identical:
function foobar () { ... }
var foobar = function () { ... };
You can still call functions set this way with parameters just like normal:
var foobar = function (str, num) { ... };
foobar('some silly string', 27);
Once you understand that, its easy to make the leap from variable to object since objects can be assigned to variables, and objects are always written with curly brackets (just like functions):
var obj = { name: "Bill", job: "cat herder" };

Andrei Fecioru
15,059 PointsWell, functions are objects in all respects and can be treated as such. The two answers before me make some very good points, but let's take a look at the following sample of JS code:
var foo = function() {...};
So far, so good: we have a function expression in which we assign to the foo
variable. Nothing special. But then, we can write:
foo.bar = "bar";
console.log(foo.bar); // this will output 'bar' at the browser console
Yes, that is right: just like any other object, you can set new properties on a function object. Obviously you can remove them if you like:
// delete the 'bar' property from the 'foo' function object
delete foo.bar;
What is this good for? This is very handy for a technique called memoisation; through such a technique you can cache the results of a very computational expensive function to be re-used later if the function is called with the same input.
Example: let's say we have a function encrypt
which receives an input string and outputs another string: the encrypted version of the input. Let's also suppose that the encryption algorithm is something that takes a lot of CPU cycles to perform. We could write the function as follows:
function encrypt(input) {
// let's setup the cache first, by setting a 'cache' property on the function itself
// (if it's not already there)
encrypt.cache = encrypt.cache || {};
// have we already computed the encryption for the input?
// if so, just return the cached result and avoid the expensive computation.
if (encrypt.cache[input]) return encrypt.cache[input];
// if not, we have to do it the hard way...
var output = ... ; // this is where the expensive encryption occurs
return output;
}
Note that this technique works if we know in advance that the set of possible inputs that we will feed the encrypt
function is limited in some manner. Otherwise, the contents of the encrypt.cache
property could grow to very large proportions. This is a classic case of space vs. speed tradeoff we do so often in the computer science world.
James K Doherty
Courses Plus Student 5,831 PointsJames K Doherty
Courses Plus Student 5,831 PointsThink of it like this, functions are objects because they can be passed around like objects. You can define a function inside of an object, so you have to look at the word 'function' with sort of a grain of salt. JavaScript is a bit different that way, a function is not exactly conceptualized the same as it is in other languages. What has helped me in understanding JavaScript is to not get too caught up in its definition of terms, it's a bit flexible in the meaning or expression of some things. Kind of like building a model of a building with popsicle sticks instead of casting one out of plastic, they're both models of buildings and will function the same way but the plastic one has a more rigidly defined material with a more specific or singular purpose. JavaScript is like the popsicle building. If I build a model building from popsicles, I can effortlessly define a bridge from the same popsicles, it would be much more work to take that plastic building and turn it into a bridge because it already has a rigid shape of a building. Does that help or did I make this more confusing? lol.