Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial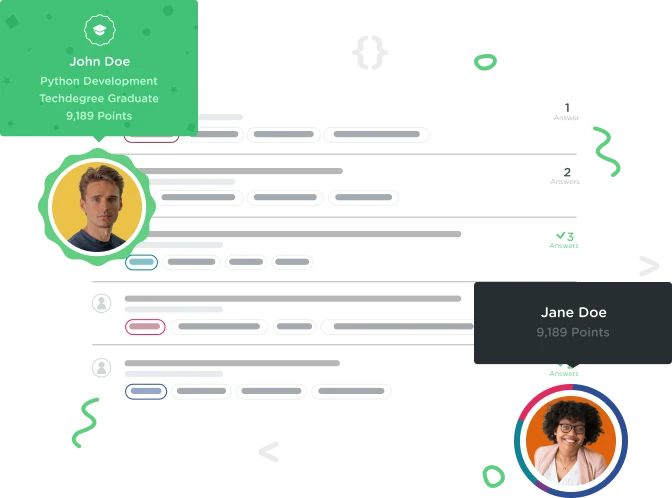
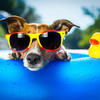
Paul Melos
3,905 PointsWhat does Kenneth mean that you can't set sneaky?
He says in the video that we've lost the ability to set sneaky...
Since Character class accepts kwargs, wouldn't you just pass it when you initialize a thief object??
like this:
obj = Thief("bill", sneaky=True)
import random
class Character:
def __init__(self, name, **kwargs):
self.name = name
for key, value in kwargs.items():
setattr(self, key, value)
class Thief(Character):
sneaky = True
def pickpocket(self):
return self.sneaky and bool(random.randint(0, 1))
def hide(self, light_level):
return self.sneaky and light_level < 10
2 Answers

CHRISTOPHER CONNOR
4,740 PointsWe want the Thief to have sneaky as an ability as standard rather than using it as an add on using **kwargs.
In the same way if we decided to create a class for a Knight character you could have sword as standard when creating the Knight and not need to add sword using kwargs.
obj = Knight("Arthur", "Excalibur")
rather than
obj = Knight("Arthur", sword="Excalibur")
I hope that makes sense like it does in my head :)
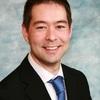
Mark Chesney
11,747 PointsHi Paul. Yes, I'm still figuring super() and OOP out myself, but you're correct -- by using super() when we initialize a Thief() or a Knight(), we reduce written code. After all, all characters have the ordinary character attributes, including name. But not all characters have a sneaky attribute, or a sword/Excalibur attribute. I think Christopher's example is a good one.
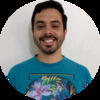
Ewerton Luna
Full Stack JavaScript Techdegree Graduate 24,031 PointsCan you tell me if I understood this right? What if I wrote my code like this (just for the sake of it)
class Thief(Character):
sneaky = True
def __init__(self, name, sneaky = True, **kwargs):
self.name = name
self.sneaky = sneaky
for key, value in kwargs.items():
setattr(self, key, value)
Let's pretend I never heard about the super() function, so I wrote my code like this. Can I say that this code does the same thing and works just as Kenneth's? If so, I come to the conclusion that super() helps you to write less code, because I don't have to repeat what I wrote in the super class (among other things I've not learned yet).
Paul Melos
3,905 PointsPaul Melos
3,905 PointsThank you Christopher, it sure does. Between your explanation and fussing around with the workspace, it is making more sense. To anybody else who needs an illustrative example, pay close attention to the ability pass "True" for sneaky before and after super() is used to override the parent classes's init method. You may have to go back to the "Methods" video where Kenneth introduces us to the init method.
Before adding the Character parent class you can initialize a thief object and set sneaky like so:
obj = Thief("Bill". True)
After adding the Character parent and removing the init method from the Thief subclass, you will get an error. I had to remind myself that this is a complicated topic and Kenneth had to figure out a simple way to introduce super(), which in itself made super() confusing because the example was so simple.
So then why use super() in real life? Still figuring that out, but I believe this has implications for DRY as your classes and subclasses become more intricate. You don't want to have to remember to pass kwargs every time you initialize a class. Also something that every answer in the googlenets mentions is Method Resolution Order, MRO which is another complex topic, but super() seems to be used to control the way your subclasses recieve or don't receive methods from thee parent classes via manipulating the MRO.
More here https://rhettinger.wordpress.com/2011/05/26/super-considered-super/