Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial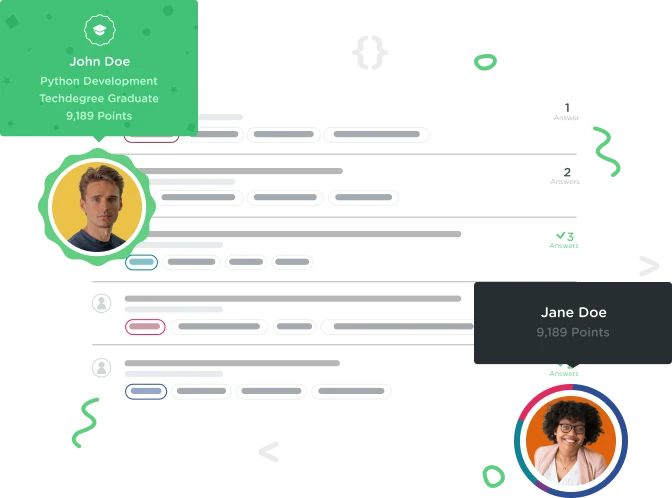

Ellis Beale
2,664 PointsWhat does "Killed" mean?
The good news: I'm no longer getting the errors we started with. The bad news: now it just says "Killed" and nothing else.
2 Answers
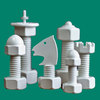
Steven Parker
231,269 PointsIn the "addNewTeachers" function you have this loop:
for (let j=0; j<teachers.length; j++) {
teachers.push(newTeachers[j]);
}
Note that each time through the loop, the "push" will add a new item to the "teachers" array, increasing the length. So no matter how many times it runs, the index number will always be smaller than the length. And since it rapidly consumes memory as this happens, the monitor process determines that it has "gone rogue" and kills it.
You probably meant to base your loop conditional on newTeachers.length instead of teachers.length.
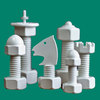
Steven Parker
231,269 PointsYou probably have a runaway (endless) loop. The workspaces have a background process that monitors how much memory other processes are taking, and how quickly they use up more. If a process starts acquiring memory quickly and it continues for too long, this monitor process will send the other one a "kill" signal to stop it. When that happens, you see the word "Killed" printed by the system to indicate why your process ended.
If you have trouble identifying why your program might be doing this, you could post the code here and perhaps someone can help you spot it.

Ellis Beale
2,664 Pointsconst teachers = [
{
name: 'Ashley',
topicArea: 'Javascript'
}
];
const courses = ['Introducing JavaScript',
'JavaScript Basics',
'JavaScript Loops, Arrays and Objects',
'Getting Started with ES2015',
'JavaScript and the DOM',
'DOM Scripting By Example'];
function addNewTeachers(newTeachers) {
// TODO: write a function that adds new teachers to the teachers array
for (let j=0; j<teachers.length; j++) {
teachers.push(newTeachers[j]);
}
}
function printTreehouseSummary() {
// TODO: fix this function so that it prints the correct number of courses and teachers
for (let i = 0; i < teachers.length; i++) {
console.log(`${teachers[i].name} teaches ${teachers[i].topicArea}`);
}
console.log(`Treehouse has ${courses.length} JavaScript courses, and ${teachers.length} Javascript teachers`);
}
const newTeachers = [
{
name: 'James',
topicArea: 'Javascript'
},
{
name: 'Treasure',
topicArea: 'Javascript'
}
];
addNewTeachers(newTeachers);
printTreehouseSummary();