Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial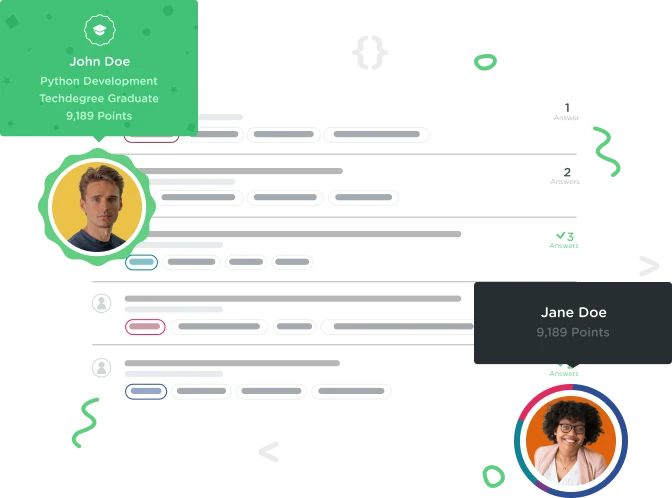

Chris Kwong
10,266 PointsWhat does "||" mean in javascript in a variable declaration? Example var variable = a || b;
I was looking at some code in a forum and this:
current = newGallery || 0;
popped up. I think it means: if newGallery is not null or undefined, set it to 0, otherwise current = newGallery.
What is this syntax? Is there an equivalent with &&. I only know of || and && as boolean OR and AND
2 Answers
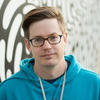
Jim Withington
12,025 PointsWell, it's my understanding that || is going to be that Boolean "or," so this is going be "set current to either the variable called newGallery or zero," I believe!
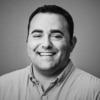
mikes02
Courses Plus Student 16,968 PointsIt's a good idea to do a test to see how this works. It's not specifically looking for 'null' or 'undefined' it's actually looking for truthy/falsey. James Padolsey has an excellent write up on Truthy & Falsey worth reading.
In JS there are 5 falsey values: undefined, null, NaN, 0, "" (empty string), and false, of course.
So in a simple test we can look at a truthy equivalent:
// Truthy
var newGallery = 1;
var current = newGallery || 'false';
alert(current); // Alert returns 1
and falsey:
// Falsey
var newGallery = 0;
var current = newGallery || 'false';
alert(current); // Alert returns false

Chris Kwong
10,266 PointsActually, you reversed it
the point is to set a default value when newGallery is null or undefined. I am just unsure of the syntax since I've only learned || as OR in a boolean statement, not in a variable declaration.
var defaultValue = 3;
var newValue = oldValue || defaultValue;
so if oldValue is null or undefined, it is supposed to be set to defaultValue of 3. But I am confused of the syntax. Is it a boolean statement now? What if oldValue is 0, is that falsey?
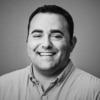
mikes02
Courses Plus Student 16,968 PointsI'm not sure what you mean by reversed, did you run the tests in your browser? I just wanted to demonstrate the truthy/falsey. If newGallery equates to a falsey value then it will alert 'false', if it doesn't, it will use the defined value. The || is still an OR operator even when used in a variable declaration. It's basically saying "do this OR that" depending on what the variable value is determined to be.
Your example would immediately set newValue = 3 because you don't have an oldValue defined anywhere, so it would be falsey. As a side note you'd also need the variable oldValue defined or else it would throw an error.
// oldValue is falsey, so newValue = 3
var oldValue;
var defaultValue = 3;
var newValue = oldValue || defaultValue;
alert(newValue);