Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial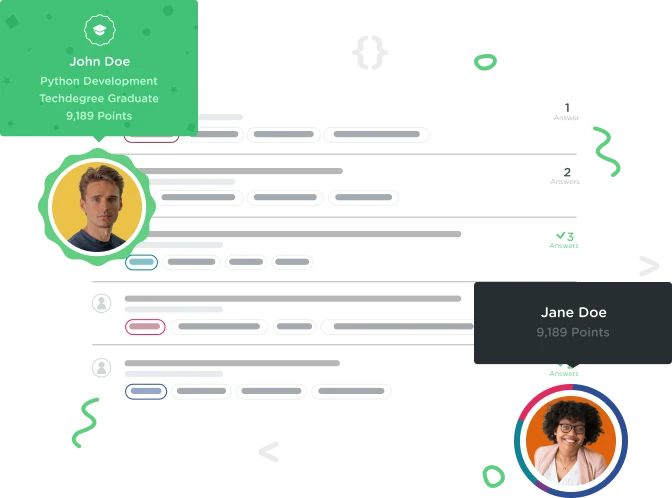

Frederick Pearce
10,677 PointsWhat does request.args.get return?
This is a comment, not a question, regarding one of the quiz questions in Flask basics. It's a good guess just from carefully reading the question that "query strings" probably return strings, but I didn't pick up on this right away, so wrote a simple test I thought I would share that proves this point:
from flask import Flask
from flask import request
app = Flask(__name__)
@app.route('/')
def index(name=""):
# for example, try ?name=1.0
name = request.args.get('name', name)
return "You entered a query string of {}, and request.args.get returned it as a {}, of course!".format(n ame, type(name).__name__)
app.run(debug=True)
1 Answer
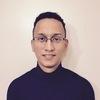
Daniel Santos
34,969 PointsI did some research and I learned that .get() is a method of the dict class. So as any dictionaries behavior, if you do:
my_dict = {'Name': 'Daniel', 'Age': 22}
name = my_dict.get('Name')
age = my_dict.get('Age')
name will be the same datatype as the data in the dictionary. I don't know if this make sense. For example, name will be a string type and age will be a integer type. I made this example using the python3 shell.
>>> dict = {'Name': 'Zara', 'Age': 27}
>>> dict.get('Name')
'Zara'
>>> dict.get('Age')
27
>>> response = dict.get('Age')
>>> response_two = dict.get('Name')
>>> response.type()
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
AttributeError: 'int' object has no attribute 'type'
>>> type(response).__name__
'int'
>>> type(response_two).__name__
'str'
That was my research, and now to respond your question. request.args.get() will always return a string. And the reason is, it has been stored as a string. Does this make any sense for you?
For example, you have this query string. http://www.mysite.com/?name=Frederick&language=Python&version=3
If you do
type(request.args.get('name')).__name__
# This will be a string.
type(request.args.get('version')).__name__
#This also will be a string
In addition, you can always cast this data types.
# String to int
# Assuming that the string are numbers
string = '34'
to_num = int(string)
# Int to string
num = 34
to_string = str(num)
I hope this help, -Dan
Daniel Santos
34,969 PointsDaniel Santos
34,969 PointsWhat was the output?