Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial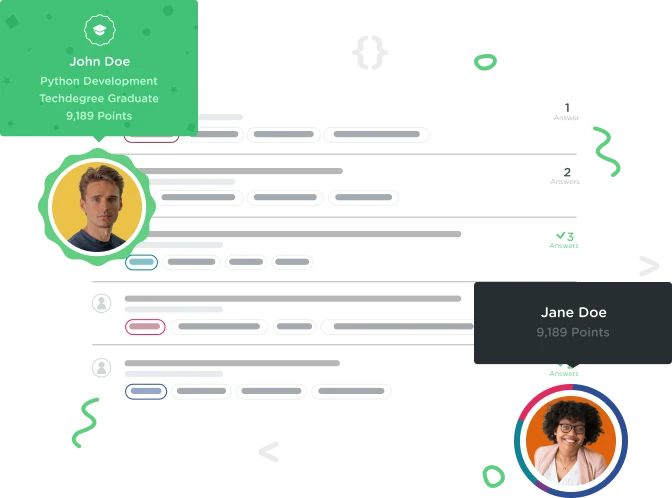
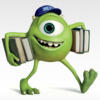
Tobias Edwards
14,458 PointsWhat does setattr() actually do?
In this series, Kenneth keeps using setattr(), but why?
What actually does this function do and how do you use it?
Thank you
1 Answer
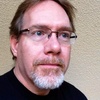
Chris Freeman
Treehouse Moderator 68,454 PointsAs stated in the docs, setattr() is the counterpart of getattr(). The arguments are an object, a string and an arbitrary value. The string may name an existing attribute or a new attribute. The function assigns the value to the attribute, provided the object allows it. For example, setattr(x, 'foobar', 123)
is equivalent to x.foobar = 123
.
setattr()
can be used when the name of the attribute you wish to set is contained in a variable.. Say you have a set of keyword variables you wish to add to an object as attributes. You can loop through the kwargs and add them using setattr()
:
$ ipython3
Python 3.4.0 (default, Jun 19 2015, 14:20:21)
Type "copyright", "credits" or "license" for more information.
IPython 1.2.1 -- An enhanced Interactive Python.
? -> Introduction and overview of IPython's features.
%quickref -> Quick reference.
help -> Python's own help system.
object? -> Details about 'object', use 'object??' for extra details.
In [1]: class myobj():
...: pass
...:
In [2]: kwargs = {'username': "snakeherder", 'email': "sherder@example.com"}
In [3]: for key, value in kwargs.items():
...: setattr(myobj, key, value)
...:
In [4]: myobj.username
Out[4]: 'snakeherder'
In [5]: myobj.email
Out[5]: 'sherder@example.com'
Tobias Edwards
14,458 PointsTobias Edwards
14,458 PointsAwesome, thanks! Now, what does getattr() mean?
Chris Freeman
Treehouse Moderator 68,454 PointsChris Freeman
Treehouse Moderator 68,454 PointsFrom the docs: getattr(object, name[, default]) Returns the value of the named attribute of
object
.name
must be a string. If the string is the name of one of the object’s attributes, the result is the value of that attribute. For example,getattr(x, 'foobar')
is equivalent tox.foobar
. If the named attribute does not exist, default is returned if provided, otherwiseAttributeError
is raised.Using
getattr(x, 'foobar')
instead ofx.foobar
has the advantage of being able to specify a default. This prevents an error when looking up an attribute that may not exist. For example,This is easier to read then having to wrap code in a
try
statement:Tobias Edwards
14,458 PointsTobias Edwards
14,458 PointsWow, cheers :)
fahad lashari
7,693 Pointsfahad lashari
7,693 PointsChris Freeman Thank you Chris for this brilliant explanation and the perfect code example! I had been struggling to understand this for a while now. Much appreciated!
Kind regards,
Fahad