Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial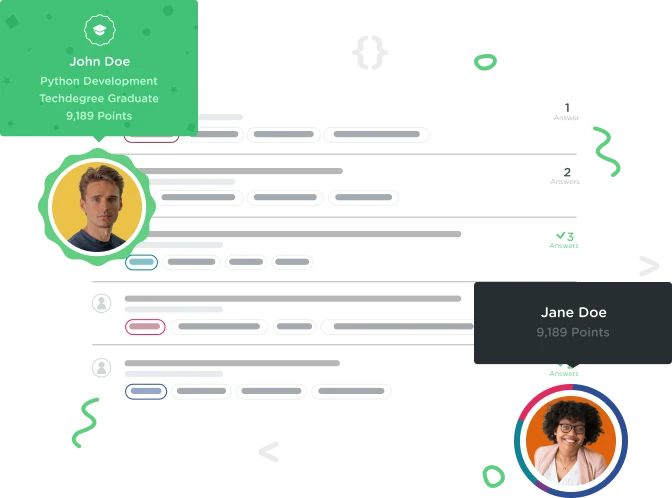

Joe Voll
304 PointsWhat does "sum += many[i]" do?
It's an increment but what exactly is it supposed to do. And why is sum+= to many[i]?
3 Answers
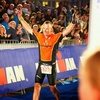
Steve Hunter
57,712 PointsHi there,
That takes the value of sum
and adds the value of many[i]
to it, and assigns that back into the value of sum
.
I assume that iterates through the array many[]
using an index of i
so that you end up with sum
having the value of all the elements of many[]
added up.
Hope that helps!
Steve.

Hayes McCardell II
643 Pointssum += many[i]
In this case, many is an array. You can tell because it has the square brackets []. The variable i is a way to access one of the indexes of that array.
So, sum += many[i] is saying, take what is currently in sum, and add whatever is inside many at index i.
It could also be written as sum = sum + many[i].
I is often used as a looping variable for a for loop. So, with this sum += code snippet inside a loop and the index starting at zero, and running until the size of the array, you would be able to sum up all the integers in the array.

Richard Then
10,690 PointsHi,
I had to watch the video several times, and go through many of the Q&As to finally understand it. I broke it down the loops into steps according to my understanding, hopefully this helps.
int many [] = {2, 4, 8};
int sum = 0;
for (int i=0; i (< 3); i++)
{sum += many [i];
printf("sum %d\n", sum); }
//Remember, *when:
0 refers to 2,
1 refers to 4,
2 refers to 8
——————————————————————————————————
Loop 1:
1a. i=0
1b. i (0) < 3
// 0 is less than 3, loop continues.
1c. sum += many[i]
// translation, everything in parenthesis.
many [i], pulls input from step 1a.
= sum (0) += many[i (*when: 0)] = new_sum_A = 2
1d. printf("sum %d\n", sum)
// “sum (new_sum_A)” = Output A: sum 2
1e. i++ = (i=0) + 1 = 1
// i=1
——————————————————————————————————
Loop 2:
2a. i=1
2b. i (1) < 3
// 1 is less than 3, loop continues.
2c. sum += many[i]
// translation, everything in parenthesis.
many [i], pulls input from step 2a.
= new_sum_A (2) += many[i (*when: 1)] = new_sum_B = 6
2d. printf("sum %d\n", sum)
// “sum (new_sum_B)” = Output B: sum 6
2e. i++ = (i=1) + 1 = 2
// i=2
——————————————————————————————————
Loop 3:
3a. i=2
3b. i (2) < 3
// 2 is less than 3, loop continues.
3c. sum += many[i]
// translation, everything in parenthesis.
many [i], pulls input from step 3a.
= new_sum_B (6) += many[i (*when: 2)] = new_sum_C (14)
3d. printf("sum %d\n", sum)
// “sum (new_sum_C)” = Output C: sum 14
3e. i++ = (i=2) + 1 = 3
// i=3
——————————————————————————————————
(non) Loop 4:
4a: i=3
4b. i (3) < 3
// 3 is not less than 3, loop stops.
——————————————————————————————————
sum 2 sum 6 sum 14
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsSo,
sum++
adds one tosum
and stores that value insum
. Usingsum += value
incrementssum
by the value ofvalue.
.