Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial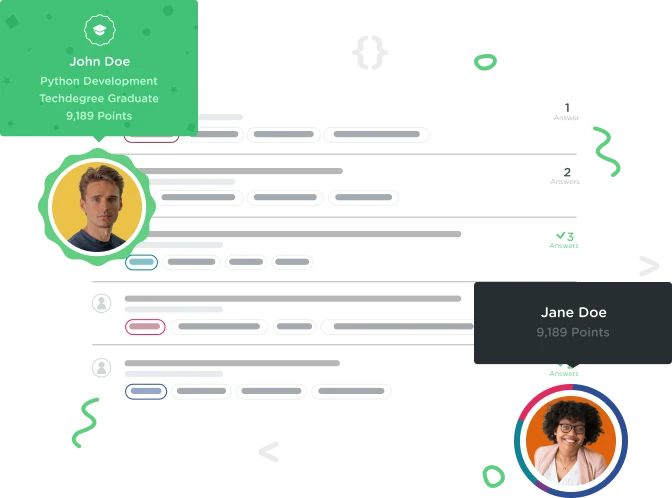

Oraseanu Daniel
1,278 PointsWhat does the exercise wants from me
I don't understand what exactly this exercise wants. I used "\s+" as regex. also used the negation of it.
package com.example;
import java.util.Date;
public class BlogPost {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
public String getWords() {
return mBody.split("[\s+ \]");
}
}
2 Answers

Mike Wagner
23,559 PointsYou're pretty close with your definition of getWords()
public String getWords() {
return mBody.split("[\s+ \]");
}
but there are a few things that need to change. First, and most importantly, since you are returning "words" you'll aren't returning just a String
but a String[]
array filled with all of the words created with the split method. If you change
public String getWords()
to
public String[] getWords()
you'll be halfway there.
The final issues rest with your regex
return mBody.split("[\s+ \]");
The \
preceding the s
needs to be escaped properly, so you'll wind up with \\s+
instead. Also, you'll need to remove the escape character in front of the closing bracket, ending with
public String getWords() {
return mBody.split("[\\s+]");
}
Now, if we changed that and run it, you'll see a different result from the challenge. It expected 11 words but it received 12. We're close. Progress! Yay us!
To put the cherry on top and finish the challenge, we need to understand that the brackets aren't exactly necessary in this case. Since we are only defining one character class \s
and it doesn't need to be separated, we could remove them for simplicity's sake. However, I like your style, so we will leave them for this exercise. The +
tells us that we are to remove 1 or more whitespace characters. Without it, we would only find the first one, then jump out.
The problem here is that we are using the brackets, which causes the +
to be matched literally as a part of the character class. If we move it outside the bracket, like so
public String[] getWords() {
return mBody.split("[\\s]+");
}
and we run the program, it will now match like we want. Challenge complete! Now...time for some real Java (coffee), haha. Good luck!

Oraseanu Daniel
1,278 PointsI understand, thanks!
Oraseanu Daniel
1,278 PointsOraseanu Daniel
1,278 PointsThank you,
It worked! Thanks for the explanations. Still there might be a problem with regex interpretation because the same expression selects different characters on an online regex interpreter.
Mike Wagner
23,559 PointsMike Wagner
23,559 PointsYeah, you'll have issues with regex pretty much anywhere you go. A lot of them are pretty good about the basics, but sometimes you'll run into some pretty interesting edge-cases. If you know what version of regex your languages (and in this case, environment as well) support, it becomes easier to mess with the tangled mess that all the various forms of regex leave us with. If you use that online interpreter for a lot of things and can't quite get it working for a few common things, it might be better to find a different one. I respect the people that make those online playgrounds, but sometimes they just don't get it 100% right.