Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial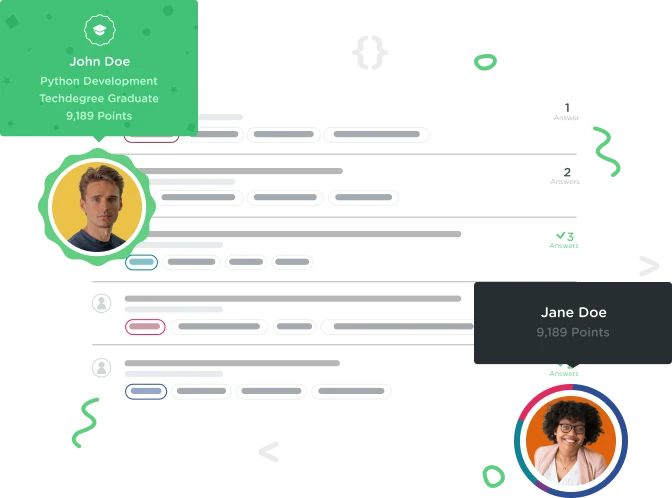

Ben Manson
5,882 Pointswhat does the pathForResource() method do when you add curly braces?
I was eventually able to solve this, in the following way:
if let plistPath = NSBundle.mainBundle().pathForResource("CrazyInformation", ofType: "plist") {
let currentCrazyInformation = NSDictionary(contentsOfFile: plistPath)
}
But I arrived at my solution through guesswork, mostly. I kept getting an error from the editor error reminding me to create an "instance" of the dictionary with the content of the plist file. In addition, I was getting an error from Swift that I had to unwrap plistPath, and it wasn't working when I just put in a bang operator at the end of the plistPath when it appears as the parameter of NSDictionary. I get that making "let plistPath" an "if let" statement does something to fix the problem with unwrapping (even though it doesn't unwrap it per se). But what does putting "let currentCrazyInformation..." in curly braces after the pathForResource method do to solve the problem? And how does putting anything in curly braces after pathForResources adjust what the method does?
import Foundation
let plistPath = NSBundle.mainBundle().pathForResource("CrazyInformation", ofType: "plist")
let currentCrazyInformation = NSDictionary(contentsOfFile: plistPath)
1 Answer
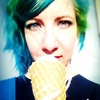
Sara Chicazul
7,475 PointsI suspect that this is a case where Treehouse's error messages are misleading. In the video, Pasan specifies that pathForResource() returns an optional, so it is safer to use the optional binding syntax, to make sure you are not trying to create your NSDictionary from a file that doesn't exist. The code challenges often implicitly expect you to use syntax as close as possible to the example given in the lesson.
The 'if let' structure checks if the value is nil and if not, lets you do something with the unwrapped value. This means code within the curly braces has access to the unwrapped value just as if you'd used the bang operator but without the chance of causing a fatal error.
In short, the braces don't affect the operation of pathForResource(). They are part of the structure the challenge expects you to use to unwrap the optional value returned by pathForResource().