Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial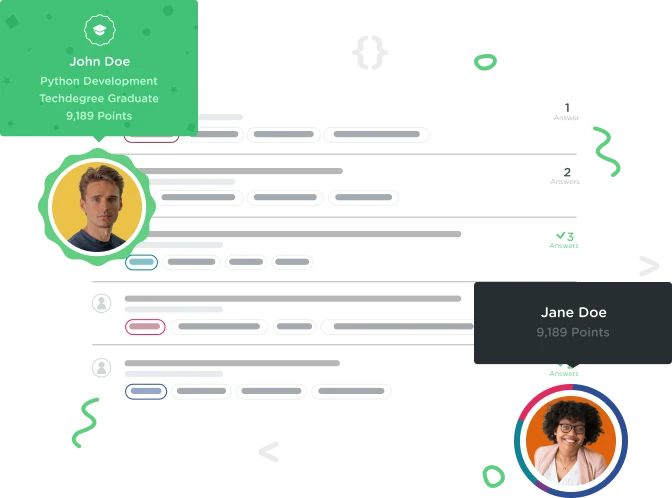
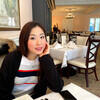
Hanwen Zhang
20,084 PointsWhat does the raw=True or raw=False mean?
def get_books(filename, raw=False): try: data = json.load(open(filename)) except FileNotFoundError: return [] else: if raw: return data['books'] return [Book(**book) for book in data['books']]
2 Answers
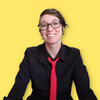
Mel Rumsey
Treehouse ModeratorHey Hanwen Zhang !
def get_books(filename, raw=False):
try:
data = json.load(open(filename))
except FileNotFoundError:
return []
else:
if raw:
return data['books']
return [Book(**book) for book in data['books']]
What this function does is it either returns a clean list of just the book titles (because the __str__
method just returns the book.title) if raw is set to False, which it is by default. Like this:
[11/22/63, Bag of bones, Carrie, Christine, Cujo, The dead zone, Dolores Claiborne, The Drawing of the Three (The Dark Tower, Book 2), Dreamcatcher, Duma Key, The eyes of the dragon, Firestarter, The girl who loved Tom Gordon, The green mile, It, Misery, Needful things, Pet sematary, 'Salem's Lot, Song of Susannah (The Dark Tower, Book 6), The dark tower (The Dark Tower, Book 7), The Shining, The stand, The Tommyknockers, The Waste Lands (The Dark Tower, Book 3), Wizard and glass, Wolves of the Calla (The Dark Tower, Book 5), The Gunslinger (The Dark Tower, Book 1)]
When it calls the function with raw=True
, it returns a list of dictionary items. (This is a long list so I am only going to copy the first couple of books)
[{'number_of_pages': 849, 'price': 13.55, 'publish_date': 2011, 'subjects': ['Time travel', 'Assassination'], 'title': '11/22/63
'}, {'number_of_pages': 732, 'price': 7.99, 'publish_date': 1999, 'subjects': ['Authors', 'Custody of children', 'Grandfathers',
'Haunted houses', 'Novelists', 'Trials (Custody of children)', 'Widowers', 'Widows', "Writer's block"], 'title': 'Bag of bones'
}, and so on........
This has all of the details for each book including num of pages, price, and subjects. Hopefully this helps a bit but don't hesitate to reach out if you are still stuck!
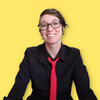
Mel Rumsey
Treehouse ModeratorHey Hanwen Zhang, when we call the function with raw=True
, it returns a list of dictionary items as mentioned above.
else:
if raw:
return data['books']
^ This section of the code kicks in and it returns the book items as a dictionary in their raw format. :)
Hanwen Zhang
20,084 PointsHanwen Zhang
20,084 PointsHi Mel, Thank you for your reply, what about if we use 'raw=True' instead of False? Thank you
Hanwen Zhang
20,084 PointsHanwen Zhang
20,084 PointsThank you so much, I understand now.