Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial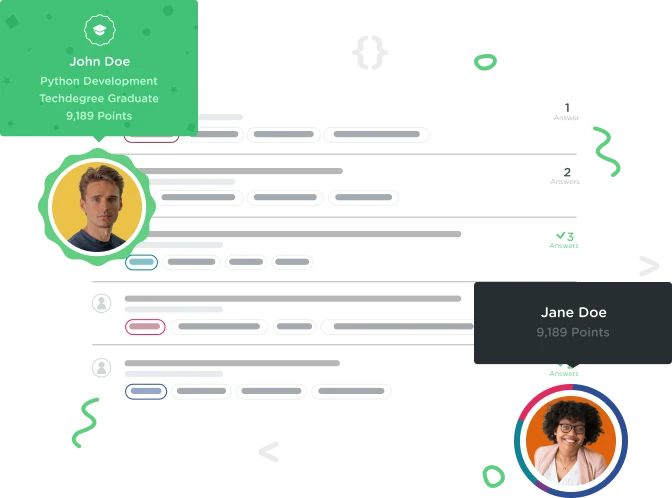
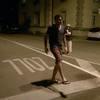
Axel Rearte
2,125 PointsWhat does the return means?
Can someone explain me what the return does? thanks.
3 Answers

David Finley
18,742 PointsThe return statement ends function execution and specifies a value to be returned to the function caller.
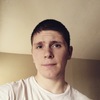
Shane May
5,114 Pointsreturn basically means output. So if you have a method that doubles numbers, and the input is 5, then the return would be ten. public int doubleNumber(int i){ return i *2; }

dman10345
7,109 PointsAs David stated above, the return statement exits out of function execution. So a return will end a loop or a method and will not execute code below it. This is great for exiting loops or logical operations based on certain conditions that are not predefined in the original loop or method or to prevent excess computations from wasting resources.
For example if you had an array of 1,000 random numbers and you needed to find the first number that was a multiple of 7 in the array, you would have to build a loop that would iterate through each item in the array because you don't know at which index the item will be. However the first item that is a multiple of 7 maybe at index 2, thus there is no need to continue with the remaining 997 iterations because you are only looking for the first instance of a number that is a multiple of 7.
Another great feature of the return statement, and its most important feature, is its ability to literally return, or give, a value back to where is was called from. For example if you built a method to check if a number was even or not you would simply check if the number you wish to check is divisible by 2 with no remainder. So it would look something like this:
private boolean checkIfEven(int num) {
return num % 2 == 0;
}
Note: The type of the method, boolean in this example, MUST always match the type of the returned value. Also every method must have a return statement, except for void methods.
So if I were to call my new method using a couple inputs I would get such
System.out.println(checkIfEven(2));
System.out.println(checkIfEven(3));
System.out.println(checkIfEven(4));
System.out.println(checkIfEven(5));
I would get the following result true false true false
Simply because 2/2 = 1 with no remainder, 3/2 = 1 with a remainder of 1 so 3%2 does not equal 0 it would equal 1, 4/2 = 2 with no remainder, and 5/2 = 2 with a remainder of 1 so 5%2 = 1.
Return values are vital so definitely play with them as they hold a huge spot in programming and will be a gaping hole later in your knowledge if you ignore them now.
Here is a simple method for checking for the first multiple of 7 in an array
//we will assume our array of integers, ranNums, has been instantiated with 1000 unknown integer values above this comment
//will return the index of the first number in the array, which is a multiple of seven
private int multipleOfSeven(int[] numArray) {
for (int i=0;i <numArray.length;i++) {
if (numArray[i] % 7 == 0) {
return i;
}
}
return -1;
}
System.out.println("The first number which is a multiple of 7 is " + ranNums [multipleOfSeven(ranNums)] + " at index " + multipleOfSeven(ranNums) + ".");
This would print out the first number which is a multiple of 7 and it's index. However this does have a small bug, if there is no number which is a multiple of 7 it will return -1, you will receive an ArrayOutOfBounds exception since -1 is not a valid index. This can be handled simply with a simple if statement or handling the return differently.