Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial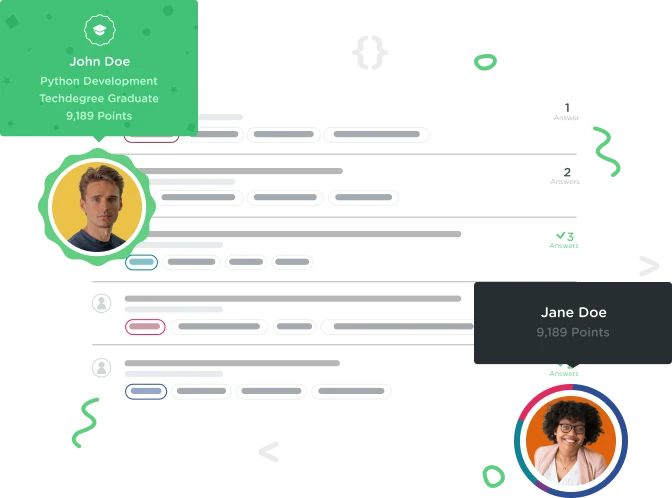
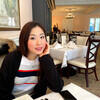
Hanwen Zhang
20,084 PointsWhat does this line ' const templateData = { id, text, name };' means in the code? Why do we use { }? what type of data?
const express = require('express');
const router = express.Router();
const { data } = require('../data/flashcardData.json');
const { cards } = data;
router.get( '/', ( req, res ) => { //redirect users to the random cards, do not need /cards since we already here
const numberOfCards = cards.length;
const flashcardId = Math.floor( Math.random() * numberOfCards );
res.redirect( `/cards/${flashcardId}?side=question` )
});
router.get('/:id', (req, res) => {
const { side } = req.query;
const { id } = req.params;
const text = cards[id][side];
const { hint } = cards[id];
if (!side) {
res.redirect(`/cards/${id}?side=question`);
}
const name = req.cookies.username;
const text - cards[id][side];
const { hint} = cards [id];
const templateData = { id, text, name }; // here
if ( side === 'question' ) {
templateData.hint = hint;
templateData.sideToShow = 'answer';
templateData.sideToShowDisplay = 'Answer';
} else if ( side === 'answer' ) {
templateData.sideToShow = 'question';
templateData.sideToShowDisplay = 'Question';
}
res.render('card', templateData);
});
module.exports = router;
3 Answers
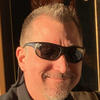
Peter Vann
36,427 PointsHi Hanwen!
In this video (around 2:20), Andrew explains rather quickly what that syntax is:
https://teamtreehouse.com/library/using-data-and-route-parameters
It is what they call "destructuring" (something new in ES6 JavaScript). It is a sort of shorthand syntax that makes extracting data out of objects (such as the video's JOSN file) easier.
This explains it well:
https://wesbos.com/destructuring-objects
Andrew references this JSON file in the video:
{
"data": {
"title": "JavaScript Flashcards",
"cards": [
{
"question": "What language are Express apps written in?",
"hint": "It starts with a \"J\"",
"answer": "JavaScript"
},
{
"question": "What is one way a website can store data in a user's browser?",
"hint": "They are delicious with milk",
"answer": "Cookies"
},
{
"question": "What is a common way to shorten the response object's name inside middleware?",
"hint": "It has the same abbreviation as \"resolution\"",
"answer": "res"
},
{
"question": "How many different values can booleans have?",
"hint": "Think: binary",
"answer": "2"
},
{
"question": "Which HTML element can contain JavaScript?",
"hint": "It starts with an \"s\"",
"answer": "<script>"
}
]
}
}
His line of code here:
const { data } = require('../data/flashCards.json');
Takes the data in the JSON file and makes it a JSON object stored in the data variable.
Then he explains that this:
const { cards } = data;
Is just an ES6 shorthand way of doing exactly this, but with slightly less code:
const cards = data.cards;
Which assigns the cards object array from the data object to the variable cards.
Destructuring is also better explained here:
https://teamtreehouse.com/library/destructuring
I highly recommend taking the entire Intro to ES6 course here:
https://teamtreehouse.com/library/introducing-es2015
...if you have not already done so.
I hope that helps.
Stay safe and happy coding!
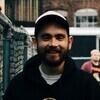
Jeffrey Holcomb
Full Stack JavaScript Techdegree Graduate 17,506 PointsHello Hanwen,
The line
const templateData = { id, text, name };
assigns a value of type Object to the variable templateData. When passing the templateData object to the pug file, you can gain access to each of the keys (id, text, and name) along with their values.

Sean Gibson
38,363 PointsThat makes sense. But then why are the variables not referenced in the cards.pug file using templateData.hint and templateData.text. Is this another feature of ES2015 that wasn't mentioned in the video?
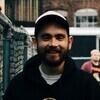
Jeffrey Holcomb
Full Stack JavaScript Techdegree Graduate 17,506 PointsSean, the variable name templateData
only exists in the cards.js file. When you pass the templateData
object into the cards.pug file, only the key/value pairs are passed. Therefore, referencing the hint
or text
values in the pug file only requires the use of their individual key names. I hope this helps!