Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial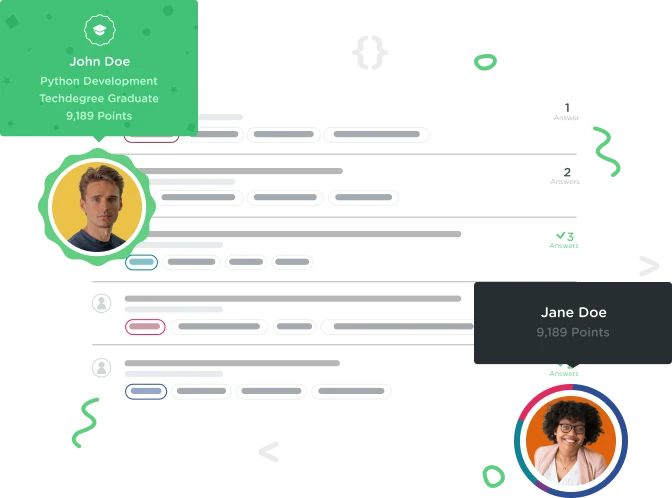
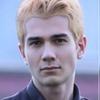
Dinu Comendant
6,049 PointsWhat happens if I don't use "console."?
so in the line of code String firstName = console.readLine(...); Craig explained the readLine, but I'm curious why we have to add "console." before that? What happens if we don't add it?
1 Answer
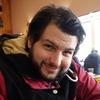
Eric M
11,546 PointsHi Dinu,
If you just use readLine()
Java will look for something to resolve readLine() to. It will expect readLine() to be a method of the current class or otherwise be in scope.
If you use it in the context of String some_variable = readLine()
then the Java compiler will also check that readLine() returns a String. There are other checks that will be performed. If you don't have a readLine() method in scope, you'll get an error that the compiler couldn't resolve the readLine()
to anything meaningful.
The real question is, why have we written console.readLine()
?
We are using an imported io library class, Console, that has line reading capability in it. You could achieve the same result with a bufferedreader or scanner class. Java programs are seperated into classes, each class generally has one or more data structures and methods to act on that data.
readLine()
is a method of the Console
class. Earlier in the program, and you can see this in the workspace, Craig has already created a Console object and he's named it console
. Java is case sensitive so there is no naming collision here.
I've put some comments in a code snippet that might help illustrate this.
// Here we import the io library's Console class
import java.io.Console;
public class Example
{
public static void main(String[] args)
{
// we create a new variable, this variable is an object of type Console
// we call this variable console. Console has been assigned System.console()
Console console = System.console();
// Here we create a new String and call it user input
// We assign whatever the readLine() method of the console object returns
// (we know that the readLine() method of the console object returns a String)
String user_input = console.readLine();
}
}
The Console object has official documentation which might be a bit of information overload, but it can be useful to look up the objects you're importing to see what else they can do and how they're expected to be used.
Happy coding,
Eric