Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial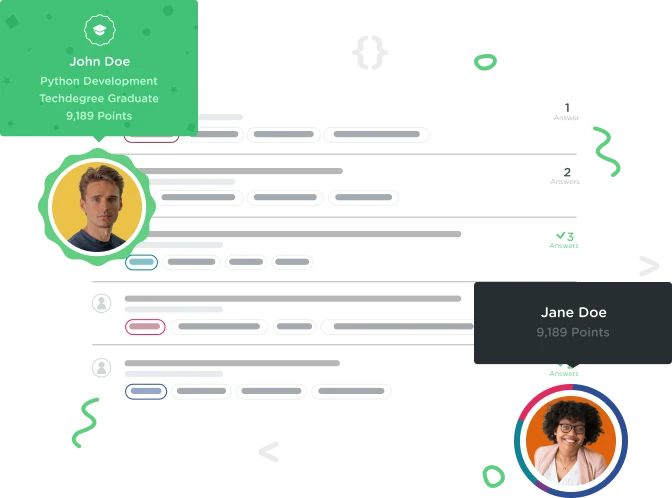

Dave Jindal
4,000 PointsWhat happens when you use the remove(int indexordata) method, in a List<int> ?
Since i assume it wouldn't be able to distinguish by using the method signature anymore :
--- "remove(int index)" and "remove(<E> data)" since <E> is now int as well ---
how is it going to decide which method to call? Is there a rule for this situation?
Like explicit signatures are prioritized over generic signatures?
2 Answers

andren
28,558 PointsHere is a quote from the Java documentation's Determine Method Signature section:
The second step searches the type determined in the previous step for member methods. This step uses the name of the method and the types of the argument expressions to locate methods that are both accessible and applicable, that is, declarations that can be correctly invoked on the given arguments.
There may be more than one such method, in which case the most specific one is chosen. The descriptor (signature plus return type) of the most specific method is one used at run time to perform the method dispatch.
The first sentence in the second paragraph is the one that seems relevant to this discussion, I interpret it as stating that Java will indeed prioritize specific type declarations over more generic ones.
I also tested this with this code:
import java.util.List;
import java.util.ArrayList;
import java.util.Arrays;
public class Main {
public static void main(String[] args) {
List<Integer> intList = new ArrayList<>(Arrays.asList(5, 0));
System.out.println(intList);
intList.remove(0);
System.out.println(intList);
}
}
Which you can run by going to this Java REPL.
Running the code results in [5, 0] being printed out first and then [0], meaning that Java interpreted the 0 argument as an index rather than a value to remove.
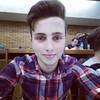
Rares Conea
Courses Plus Student 15,000 PointsYou can t make an List and declare int in the <> because int is a primitive type.None primitive type can be put between <> when declaring a list.You can put the wrapper class of int which is Integer and when you are using remove you must think what you are giving as a parameter.If you are giving and int, this means you just write remove(1) then the element in position 1 will be removed.If you write remove(new Integer(1)) then the elements with value 1 will be removed(if it exists)