Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial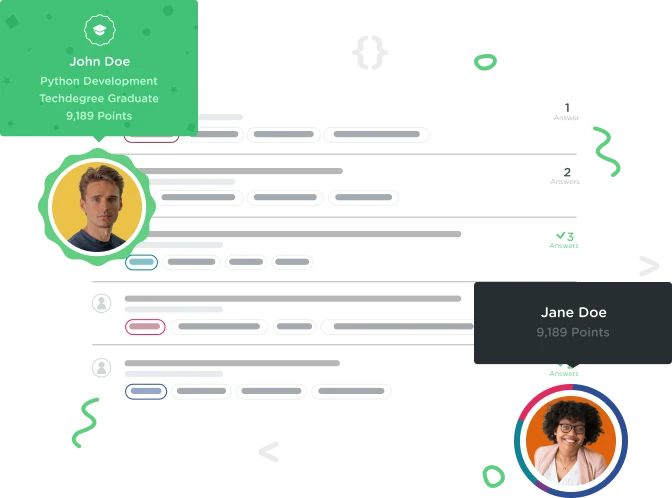

Andrei Oprescu
9,547 PointsWhat have I done wrong in my code?
Hi!
I am currently doing a challenge which has a question like this:
Our game's player only has two attributes, x and y coordinates. Let's practice with a slightly different one, though. This one has x, y, and "hp", which stands for hit points. Our move function takes this three-part tuple player and a direction tuple that's two parts, the x to move and the y (like (-1, 0) would move to the left but not up or down). Finish the function so that if the player is being run into a wall, their hp is reduced by 5. Don't let them go past the wall. Consider the grid to be 0-9 in both directions. Don't worry about keeping their hp above 0 either.
The code that I am using is at the bottom of the question.
Why Is my code wrong and how could I improve?
Thanks!
Andrei
# EXAMPLES:
# move((1, 1, 10), (-1, 0)) => (0, 1, 10)
# move((0, 1, 10), (-1, 0)) => (0, 1, 5)
# move((0, 9, 5), (0, 1)) => (0, 9, 0)
def move(player, direction):
x, y, hp = player
dx, dy = direction
if x == 0:
if dx == -1:
hp -= 5
else:
x += dx
elif x == 9:
if dx == 1:
hp -= 5
else:
x += dx
elif y == 0:
if dx == -1:
hp -= 5
else:
y += dy
elif y == 9:
if dx == 1:
hp -= 5
else:
y += dy
return x, y, hp
2 Answers
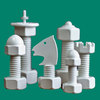
Steven Parker
229,732 PointsIt looks like you have a good basic idea, but your implementation assumes that the motion will always be a single unit (plus or minus) in one or both directions.
The instructions don't say explicitly, and none of the examples show it, but perhaps the motions they test with include some that move farther than one unit.

Andrei Oprescu
9,547 PointsThanks!
Your advice on how to fix my code worked.
I used a code like this:
def move(player, direction):
x, y, hp = player
dx, dy = direction
if x + dx < 0:
hp -= 5
elif x + dx > 9:
hp -= 5
else:
x += dx
if y + dy < 0:
hp -= 5
elif y + dy > 9:
hp -= 5
else:
y += dy
return x, y, hp
Thank you for also showing me how to format my code in a question.
Andrei
Andrei Oprescu
9,547 PointsAndrei Oprescu
9,547 PointsHi again!
I have followed your instructions and I came up with this code:
def move(player, direction): x, y, hp = player dx, dy = direction if x + dx is False: hp -= 5 else: x += dx elif x + dx > 9: hp -= 5 else: x += dx elif y + dy is False: hp -= 5 else: y += dy elif y + dy > 9: hp -= 5 else: y += dy return x, y, hp
When I run this code I gives me:
Bummer! Try again!
Does this mean I have typed something incorrectly?
Thanks!
Andrei
Steven Parker
229,732 PointsSteven Parker
229,732 PointsUse the instructions for code formatting in the Markdown Cheatsheet pop-up below the "Add an Answer" area.
Or watch this video on code formatting.
But even unformatted, I spot a few issues:
< 0
?