Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial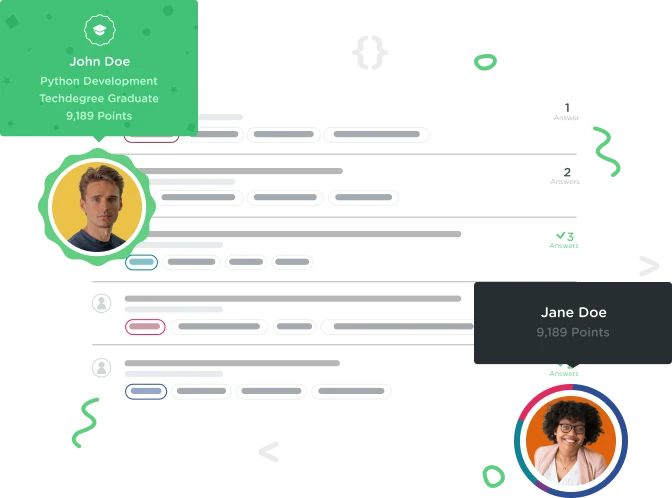
1 Answer
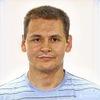
Alexander Nikiforov
Java Web Development Techdegree Graduate 22,175 PointsFirst of classes names in Java should start with Capital letter.
So rename
-
game.java
toGame.java
-
prompter.java
toPrompter.java
In the same manner please rename all usages of Game as well
in Prompter:
import java.io.Console;
// public class prompter { // is wrong
public class Prompter {
// incorrect
// private game mGame;
// should be
private Game mGame;
// incorrect
//public prompter(game Game) {
// mGame = Game;
//}
// should be:
public Prompter(Game game) {
mGame = game;
}
public boolean promptForGuess() {
Console console = System.console();
// just an advice : never name variable "promptt", this is
// very bad convention, better would be first use "prompt"
// then "lowerCasedPrompt"
String prompt = console.readLine("Enter a letter: ");
String lowerCasedPrompt = prompt.toLowerCase();
char guess = lowerCasedPrompt.charAt(0);
return mGame.guess(guess);
}
public void display() {
System.out.printf("to solve: %s \n", mGame.getProgress());
}
}
The same goes to Game
class
// public class game { // wrong
public class Game {
private String mAnswer;
public static final int MAX_TRIES = 7;
// public int hits = 0; // don't make them public, use getters
// public int miss = 0; // don't make them public, use getters
// initialization is better to be put in constructor
// also it should be obviously named "misses" not "miss"
// that is misleading.
private int misses;
private int hits;
// getters for non-static members
public int getHits() {
return hits;
}
public int getMisses() {
return misses;
}
// public game(String answer) { // wrong
public Game(String answer) {
mAnswer = answer;
// initialization of members
misses = 0;
hits = 0;
}
public boolean guess(char letter) {
boolean isHit = mAnswer.indexOf(letter) >= 0;
// if (isHit == false) { // not conventional
if (!isHit) {
misses += 1;
} else {
hits += 1;
}
return isHit;
}
public String getProgress() {
String progress = "";
for (char letter : mAnswer.toCharArray()) {
char display = '-';
// if (hits.indexOf(letter) >= 0) { // wrong
if (mAnswer.indexOf(letter) >= 0) {
display = letter;
}
progress += display;
}
// missing return statement
return progress;
}
}
And finally Hangman
public class Hangman {
public static void main(String[] args) {
// Enter amazing code here:
// game Game = new game("Lucia"); //wrong
Game game = new Game("Lucia");
// prompter Prompter = new prompter(Game); // wrong
Prompter prompter = new Prompter(game);
// in Java never make variable name start from capital letter
// only classes names start with Capital Letter
// boolean GameOver = false; // wrong
boolean gameOver = false;
// Prompter.display(); wrong
prompter.display();
// while (GameOver == false) { // wrong
while (!gameOver) { // in Java booleans are not compared using "=="
// boolean isHit = Prompter.promptForGuess(); // wrong
boolean isHit = prompter.promptForGuess();
// int triesleft = Game.MAX_TRIES - Game.miss; // wrong
// only "static" members can be accessed directly
// like Game.MAX_TRIES
// non-static members like "misses" should be accessed using
// getters : i.e. getMisses();
// use Camel-Case so "triesLeft" not "triesleft"
int triesLeft = Game.MAX_TRIES - game.getMisses(); //
if (triesLeft == 0) {
System.out.printf("game over \n");
// GameOver = true; // wrong
gameOver = true;
}
if (isHit) {
System.out.printf("We got a Hit \n");
// Prompter.display(); // wrong
prompter.display();
} else {
System.out.printf("We got a Miss \n");
System.out.printf("%s tries left \n", triesLeft); // change to camelCase
// Prompter.display();
prompter.display();
}
}
}
}
Here are mostly syntax errors. I didn't check the logic, but I've seen that Game yet does not work properly, but that I'll leave for you to fix.