Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial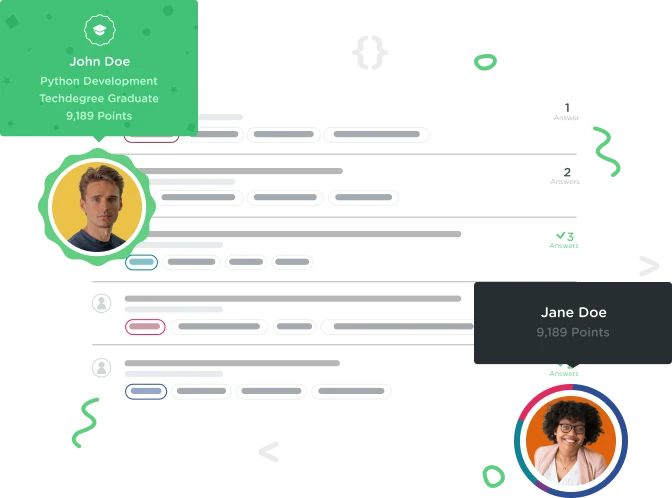

Hugo M
4,517 PointsWhat if a string is in the array more than once?
Hi guys, I was wondering what you'd have to do if the string was in the array more than once? Say apple was at position 0 and again at 5.
The indexOf function gives up after if finds the first instance. The only way I can think of doing it is by looping the function until it returns -1. Thereby returning all the instances of apple. Is there an easier way?
3 Answers
William Li
Courses Plus Student 26,868 PointsHi, Hugo. The complete syntax of the indexOf() method is string.indexOf(searchValue, startingIndex)
, the 2nd argument startingIndex is optional, by default, if you don't pass in the 2nd argument to indexOf() method call, it starts its search from the beginning of the String, and return the first occurrence of the search value.
If there're multiple occurrences of the search value in a String, and you'd like to catch them all. You can just write a loop, find the first occurrences of the word using string.indexOf(searchValue)
, then continue the search by calling string.indexOf(searchValue, foundIndex + 1)
until it eventually exhausts all the possible occurrences of the search value in the given string and returns -1.
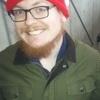
Christian Weatherford
8,848 PointsYou're right that looping is the best option, but simply calling array.indexOf("apple") will just give you the the first occurrence over and over. You'll want to use the form of the indexOf method that takes a starting point as well. So something like:
var array = [/*contents of array*/];
var searchString = "apple";
var index = -1;
do {
index = array.indexOf(searchString, index + 1);
if (index !== -1) {
console.log("The string " + searchString + " was found at index " + index);
}
} while (index !== -1);

Hugo M
4,517 PointsWilliam Li Christian Weatherford
Cheers guys :)