Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial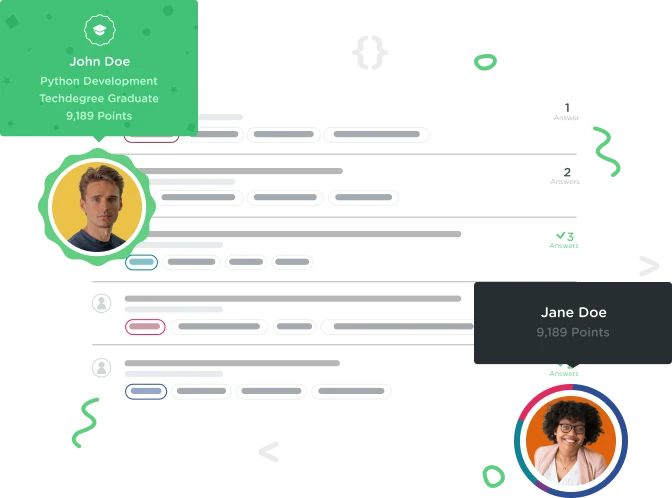

Montalvo Miguelo
24,789 PointsWhat if I have Users Table ?
I have added Users migration to my example, a to-do has user_id. (like we did in todo-item, has todo_list_id)
I'm trying to create to-do lists for users...
<?php
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Database\Migrations\Migration;
class CreateTodoListsTable extends Migration {
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('todo_lists', function(Blueprint $table)
{
$table->increments('id');
$table->integer('user_id');
$table->string('name')->unique();
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::drop('todo_lists');
}
}
<?php
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Database\Migrations\Migration;
class CreateTodoItemsTable extends Migration {
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('todo_items', function(Blueprint $table)
{
$table->increments('id');
$table->integer('todo_list_id');
$table->string('content')->unique();
$table->dateTime('completed_on')->nullable();
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::drop('todo_items');
}
}
<?php
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Database\Migrations\Migration;
class CreateUsersTable extends Migration {
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('users', function(Blueprint $table)
{
$table->increments('id');
$table->string('name', 100);
$table->string('email')->unique();
$table->string('password');
$table->rememberToken();
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::drop('users');
}
}
With routes, at the beginning we had :
<?php
Route::resource('todos.items', 'TodoItemController', ['except' => ['index', 'show']]);
If I now I have users, how the routes would be?
users/{users}/todos/{todos}/items/create
<?php
Route::resource('users.todos.items', 'TodoItemController', ['except' => ['index', 'show']]);