Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial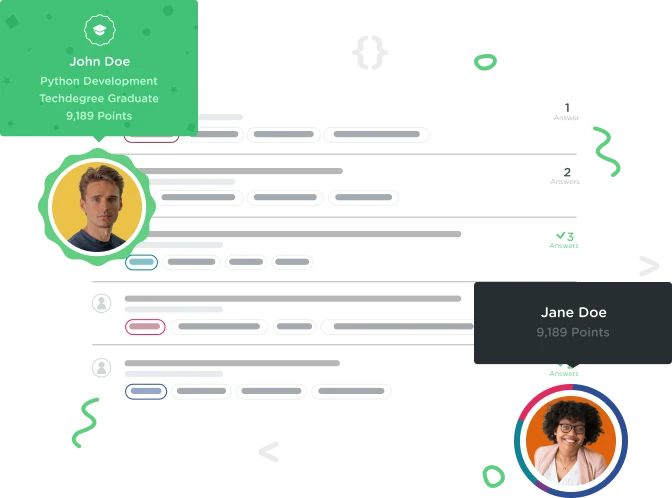
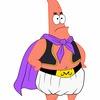
<noob />
17,062 PointsWhat if i want to make a method that pick a different word from an array in a random order when i start the game?
public static String randomWord()
{
String[] possibleWords = {"treehous", "coding", "javaisfun"};
Random random = new Random();
int randomIdx = random.nextInt(possibleWords.length);
}
2 Answers
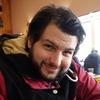
Eric M
11,545 PointsThis looks fine. What problem are you having?
If you're asking how you go from the snippet you posted to a program that, for example, prints out a random word, you'll need to use the random index you generated to access the array, then use the string at that index as you would any other. The random object also won't work unless you import Random from the java.utils library of course.
For example:
import java.util.Random;
class Main
{
public static void main(String[] args)
{
System.out.println(randomWord());
}
public static String randomWord()
{
String[] possibleWords = {"treehous", "coding", "javaisfun"};
Random random = new Random();
int randomIdx = random.nextInt(possibleWords.length);
return possibleWords[randomIdx];
}
}
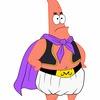
<noob />
17,062 PointsHi eric, somehow it didnβt format it? i edited it now. thanks for ur time to answer , but i didnβt meant to create a program that do that, i just wanted to add an option to randomly give the user a different word every time he launch the game, instead of the way that craig used. how do i add the randomword method to the game? , i need to use this method on my game class, and then in the hangman file i need to write to call the method instead of passing the string βtreehouseβ? and another question is why u made this method static? i will appreciate ur help Eric McKibbin
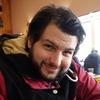
Eric M
11,545 PointsHi noob,
I kept it static from the example code you posted. A static method can be called without instantiating a class object.
This is a simple method* that returns a string. You can use this method as you would any other. So you say you want to give the user a random word every time they start the game - when they start the game now there must be a String somewhere, you replace the String with a call to this method (which returns a string).
I would encourage you to complete the Beginning Java track before trying to make these changes. A solid understanding of the fundamentals will prevent a lot of wasted time.
*I should also say that it's bad practice to have the possibleWords array hard coded to the method like this. It would be better if randomWord() had took an argument (e.g. randomWord(String[] wordList)
) and returned a string from within the passed list of words. This makes it more flexible and also means you don't have to change the method to change the words list.
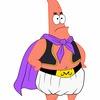
<noob />
17,062 PointsEric McKibbin Hi, thanks for ur reply :D, i understand that in order to do it i need to continue the course(which i will :P), but can u check my logic and some question of mine?
ok first all i created a new method
public String randomWord(String[] wordList)
{
Random random = new Random();
int randomIndexWord = random.nextInt(wordList.length);
return wordList[randomIndexWord];
}
now i started to get confused, i have the the "answer" variable and the String [] wordList, i cant figure out how i will check the equality, if the word in the wordList is the answer and ? >> do i even need to make an if statement?
after i pass this struggle i need to call a different word everytime the user begins a game so i switched to the HANGMAN.java file which contains the main class and its the executable file, i tried to do it in this way and it didnt worked(in the game object file i declared a parameter that contains an array of words so passed 2 words when i called the method, is it the right approach? , what about the "answer" variable? that is why i cant figure out yet :P:
public static void main(String[] args)
{
// Your incredible code goes here...
Game game = new Game(randomWord({"treehouse", "testing"}));
}
I know after i complete the track i will have more understanding of this things but i still wanted to try XD. i will appreciate any insights from u, i know i asked alot of things :|
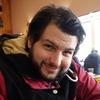
Eric M
11,545 PointsHi noob,
Your missing some understanding of the Java language and the structure of Java programs which would allow you to complete this. The reason I suggest you go through the Beginning Java (and ideally also Intermediate Java) before trying to do this is because those tracks will explain what you need to know a lot better and in a lot more depth than I can sitting here typing up an answer.
Regarding your answer comparison, String equality in java is tested with the .equals String method, however the video https://teamtreehouse.com/library/determining-if-the-game-is-won, a few videos in from the one that you linked shows how in this game you should be checking the isWon() method of the game class.
I appreciate that you're trying to learn by doing and I think it's a great approach, but a lot of what you're asking will be clearer once you've gone through a bit more of the great material here on Treehouse.
Cheers,
Eric
Eric M
11,545 PointsEric M
11,545 PointsBy the way, you want 3 backticks for code formatting.
e.g.
```Java
your code here
```