Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial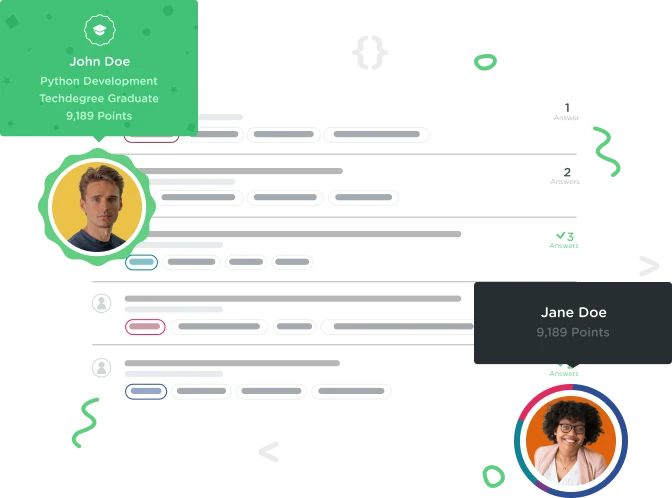
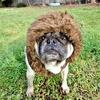
Kathryn Kassapides
9,260 PointsWhat if I wanted it to turn a different color each time I clicked on it?
I tried this but it didn't make the words change from black to purple to red because it just turned red: const myheading = document.getElementById('myHeading');
myHeading.addEventListener('click', () => {
myHeading.style.color = 'purple'; myHeading.style.color = 'red'; });
2 Answers
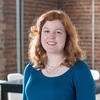
Sharon Hartsell
4,403 PointsJavaScript is changing the text color to purple, then immediately changing it again to red. If you want the text to change to purple, stay like that for a bit, then change to red, you need to add a setTimeout function to tell JavaScript to wait before changing the color.
myHeading.addEventListener('click', () => {
// Text turns purple as soon as the user clicks it...
myHeading.style.color = 'purple';
window.setTimeout(() => {
// ...and 2 seconds later, it changes to red
myHeading.style.color = 'red';
}, [2000]); // 2000 is the number of milliseconds to wait before running the code inside setTimeout
});

Jordan Davis
7,696 PointsThis is how i got it to change to a random color after each click.
const myHeading = document.getElementById('myHeading');
myHeading.addEventListener('click', () => {
myHeading.style.color = randomRGB(randomValue);
});
function randomRGB(value) {
const color = `rgb(${value()}, ${value()}, ${value()})`;
return color;
}
const randomValue = () => Math.floor(Math.random() * 256);