Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial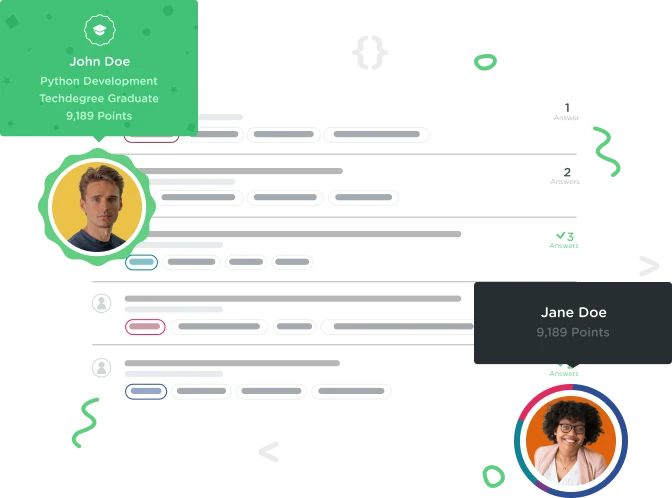

Jeremy Barbe
8,728 PointsWhat if I wanted my answers to be strings?
This code only works if the answers to the questions are numbers, and not strings. I attempted to figure out a solution on my own, but unsurprisingly it didn't work out. I basically tried changing the answer variable to parseInt(questions[i][1]) and thought since the response variable had been parsed as well, they would come up even and the loop would function as it should (parseInt sort of confuses me, probably completely confused). Any help?
var questions = [
['How many states are in the United States?', 50],
['How many continents are there?', 7],
['How many legs does an insect have?', 6]
];
var correctAnswers = 0;
var question;
var answer;
var response;
function print(message) {
document.write(message);
}
for (var i = 0; i < questions.length; i += 1) {
question = questions[i][0];
answer = questions[i][1];
response = prompt(question);
response = parseInt(response);
if (response === answer) {
correctAnswers += 1;
}
}
html = "You got " + correctAnswers + " question(s) right."
print(html);
9 Answers

Jeremy Barbe
8,728 PointsIf I answer a question correctly, it does not register on the correctAnswers variable. No matter what I enter (in the case that the answers are strings instead of numbers) it prints on the page as zero correct. EDIT: in the case that the answers to the questions are strings, and not numbers.

Jeremy Barbe
8,728 PointsWell, all the questions in the quiz are currently limited to ones that can be answered with a number. I just want to know what I would have to do to open it up so that someone could potentially write a question that requires a word answer instead of a number.

Akilah Jones
18,397 PointsSo, are you using literal quotes in the prompt? If so, see updated answer.

Jeremy Barbe
8,728 PointsDo I just get rid of parseInt?

Akilah Jones
18,397 PointsYou don't have to get rid of it....but it's also not necessary if you use the "==" instead of "==="
I'm assuming that you are working in the web browser and entering "50" instead of 50....
Is that correct? If so, then that would be the cause of your issue.

Christian Ebner
12,132 PointsHi Jeremy,
I'm not 100% sure what your issue is here. Maybe you could specify the question.
I changed the code a little to reflect that you can use strings as well.
If you want text to be accounted for in the user's answers - an approach would be to use an if statement that checks for the values that you want to have entered. to make it irrelevant how the user writes out the string (FIfty Fifty FIFTY fifty) I added in the .toLowerCase() Remark: I did not refactor so you can see the differences at glance - you can refactor (clean up) the code quite a bit.
I added some logging code that may help you as well: console.log( "response is: " + typeof response );
Keep in mind that this is a very minimalistic example and as soon as you are going bigger, you might want to change the code to use an array or objects to translate a lot of stuff to numbers.
<script>
var questions = [
['How many states are in the United States?', '50'],
['How many continents are there?', '7'],
['How many legs does an insect have?', '6']
];
var correctAnswers = 0;
var question;
var answer;
var response;
function print(message) {
document.write(message);
}
for (var i = 0; i < questions.length; i += 1) {
question = questions[i][0];
answer = parseInt(questions[i][1]);
response = prompt(question);
console.log( "answer is: " + typeof answer );
console.log( "response is: " + typeof response );
if (response.toLowerCase() === "fifty") {
response = "50";
} else if (response.toLowerCase() === "seven") {
response = "7";
} else if (response.toLowerCase() === "six") {
response = "6";
}
response = parseInt(response);
if (response === answer) {
correctAnswers += 1;
}
}
html = "You got " + correctAnswers + " question(s) right."
print(html);
</script>```

Jeremy Barbe
8,728 PointsI just want to know how to set it up so I can ask a question like "What state do you live in?" with the answer being "Arizona", as well as have questions with numerical answers. This does help me understand the problem a bit though, thank you. I was very confused with the nature of parseInt.

Akilah Jones
18,397 PointsJeremy Barbe Please note, that even changing the "answer" in the questions array to a string won't resolve your issue if you're actually typing "50" into the prompt...
Best Regards

Jeremy Barbe
8,728 PointsI'm aware. I want the answer in the questions array to be a string, and I want the user to type that string into the prompt, and it to register as a correct answer.

Akilah Jones
18,397 PointsWell if you want the answer to be a string then you don't want to parse the response into an int....
You still haven't answered if you're typing 50 or "50" into the prompt. That would make a world of difference.

Jeremy Barbe
8,728 PointsI am not putting quotes in the prompt

Akilah Jones
18,397 PointsSo are you putting quotes around the answer?
I assumed you were using the code that you initially posted, but am not sure after your last response (re: "I want the answer in the questions array to be a string")
If your answer is indeed a string then you don't want your response to be an int (i.e. you don't want to use parseInt).

Jeremy Barbe
8,728 PointsOkay, cool. Should I still be processing the answers that are in fact integers differently? So if some answers are 50 and some are "Arizona", should they go through different pipelines? (sorry you've had to respond so many times)

Akilah Jones
18,397 PointsIf you want to use a mix of strings and integers as answers then use "==" instead of "===" (no parseInt required)
For example:
var questions = [
['How many states are in the United States?', '50'],
['How many continents are there?', 7]
];
var correctAnswers = 0;
var question;
var answer;
var response;
function print(message) {
console.log(message);
}
for (var i = 0; i < questions.length; i += 1) {
question = questions[i][0];
answer = questions[i][1];
response = prompt(question);
if (response == answer) {
correctAnswers += 1;
}
}
html = "You got " + correctAnswers + " question(s) right."
print(html);
The following lines are the key:
response = prompt(question);
if (response == answer) {
Best Regards

Jeremy Barbe
8,728 PointsThanks for your help.

Akilah Jones
18,397 PointsGlad I could help .......finally :-)
brief explanation:
"===" compares the type, then the value
e.g. '4' === '4' => true
e.g. '4' === 4 => false
"==" converts the type, then compares the value
e.g. '4' == '4' => true
e.g. '4' == 4 => true
"parseInt('string')" converts string to int
e.g parseInt('4') => 4
"parseInt(int)" returns the int
e.g parseInt(4) => 4
Akilah Jones
18,397 PointsAkilah Jones
18,397 PointsWhy would you want it as a string instead of a number?