Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial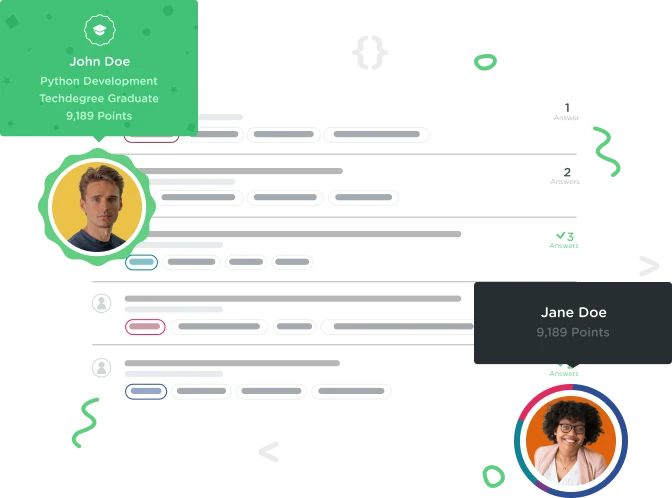
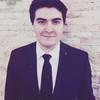
MIK 7
Courses Plus Student 881 Pointswhat if I wanted to remove multiple items in one code?
my_list = [1, 6, 9] let's remove 6 & 9, how would you do it? in one Code? solution ?
3 Answers

Jon Mirow
9,864 PointsHi there!
Short answer:
filtered_List = [ x for x in my_list if (x != 6 and x != 9)]
if there were many things you wanted removing:
things_to_remove = [3, 9, "Neighbours cat"]
filtered_list = [x for x in my_list if x not in things_to_remove]
Explaination below if desired :)
I think what we're talking about is really filtering a list right? Probably the most common way to build a new list in this kind of way is with list comprehension (we're usually building a new list, even if it's just a clone with [:], because we never want to iterate through the thing we're changing)
So ,say I wanted to make a list of 10 random numbers between 1 and 10:
from random import randrange
random_List = [ randrange(1, 11) for x in range(10)]
Okay, if you've not seen list comprehension before that looks pretty complicated right? Basically all we're doing is a normal for loop:
random_List = []
for x in range(10):
random_List.append(randrange(1, 11)
But we condense it all into one line, by wrapping it in the list brackets "[ ... ]" and putting what we want python to do before the for loop. It might look a bit weird, but this is actually closer to the english language way of describing what's happening - this is basically saying "give me a random number between 1 and 10 for every element in ...."
Just like for loops, we can add conditionals to this syntax. So to build a list comprehension that would filter out 6 and 9:
filtered_List = [ x for x in random_List if (x != 6 and x != 9)]
This one is actually a little more readable right? build a new list of each element from the old list only if that element is not 6 or 9.
List comprehension is the most common way to be honest. However you can also use filter:
even_List = list(filter( lambda x: x % 2 == 0, random_List))
Here we get a list only containing the even elements of the original random_List. Of course you could pop in the logic from the previous example to filter by 6 and 9, I'm just showing how you could use it.
The way filter works is to take a function as the first argument, an iterable as the second, and go through the iterable applying the lambda function, just like a for loop. It returns a filter object, so we have to wrap it in the list() constructor to get a list back
Obviously I can't fully explain all the code here, but I hope it helps :)
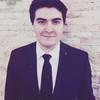
MIK 7
Courses Plus Student 881 Pointsthank you

Keith Ostertag
16,619 PointsI'm a beginner as well, but I think another way would be to use a slice (which hasn't yet been introduced in the video series at this point).
>>> my_list = [1, 6, 9]
>>> new_list = my_list[:1]
>>> print(new_list)
[1]
That gives the first member of your list. Using slice sequences can be tricky and of course this is just a very simple example.