Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial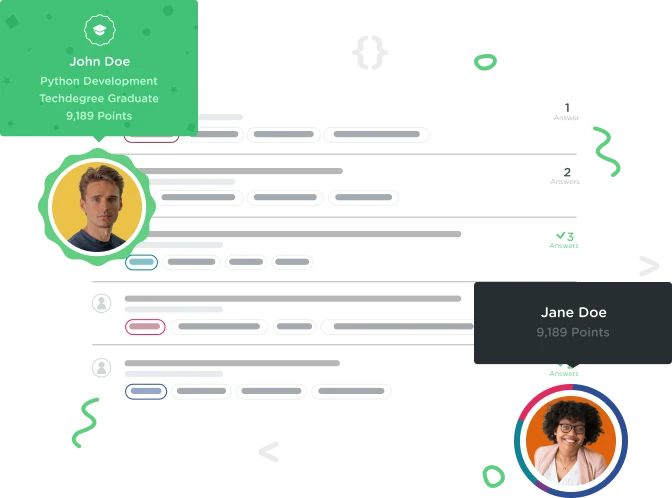
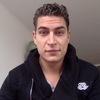
Berry Loeffen
4,303 PointsWhat if there is no initial value for numberOfSeats?
Hi there,
Been trying to do some practice with initializing subclasses. What if the numberofseats constant did not have a value already when writing the property? How would i Have to write the code? property self.numberOfSeats not initialized at super.init call is the error i get. I have to initialize numberOfSeats, i get that, but whatever I try Xcode keeps coming up with an error
class Vehicle {
var numberOfDoors: Int
var numberOfWheels: Int
init(withDoors doors: Int, andWheels wheels: Int) {
self.numberOfDoors = doors
self.numberOfWheels = wheels
}
}
class Car: Vehicle {
let numberOfSeats: Int
override init(withDoors doors: Int, andWheels wheels: Int) {
super.init(withDoors: doors, andWheels: wheels)
}
}
2 Answers
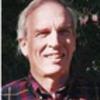
jcorum
71,830 PointsClose. You need the initializer to accept a formal parameter for the number of seats. Here I've called it seats. Because this init is now not overriding the super class's init, you can't say override. When you call this init you need to call it with 3 values. Then, with the seats value you initialize the sub class's member variable, and with the other two you initialize the inherited member variables by calling super.init(), as you were doing.
class Car: Vehicle {
let numberOfSeats: Int
init(withDoors doors: Int, andWheels wheels: Int, seats: Int) {
numberOfSeats = seats
super.init(withDoors: doors, andWheels: wheels)
}
}
If you wanted to be able to create Cars without specifying a number of seats you could add a second init, one which does override the superclass's init:
override init(withDoors doors: Int, andWheels wheels: Int) {
numberOfSeats = 1
super.init(withDoors: doors, andWheels: wheels)
}
Then you would create your Cars in two different ways:
let c1 = Car(withDoors: 4, andWheels: 4)
let c2 = Car(withDoors: 2, andWheels: 4, seats: 2)
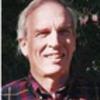
jcorum
71,830 PointsOne method overrides another if it has the same name, same method signature (number and type of parameters) and is in a different (sub) class. I think of the double r in override as "replacing or revising" the inherited method.
Since it's easy to confuse override and overload, I think of the l in overload as layering: layering on methods with the same name, different method signatures, all in the same class.
Berry Loeffen
4,303 PointsBerry Loeffen
4,303 PointsCorrect me if i'm wrong, so we only override the init method if the superclass's and subclass's init are the same? Or offcourse if there is a new stored property that already has a value. If not we create a new init.
Thanks for the help!