Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial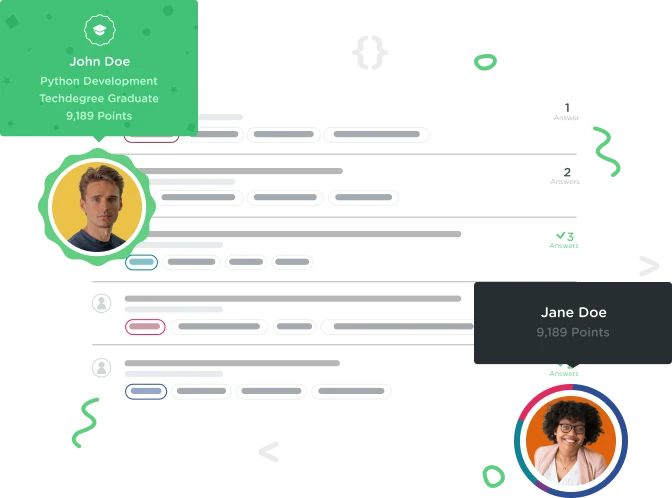

anthony amaro
8,686 Pointswhat if we don't give any parameters to the function?
in line 14 dave created a function printList( list ). i wrote it again , trying to figure out how it works and got the same result doing it this way.
function print(message) {
document.write(message);
}
function printList() {
var listHTML = '<ol>';
for (var i = 0; i < playList.length; i ++) {
listHTML += '<li>' + playList[i] + '</li>';
}
listHTML += '</ol>';
print(listHTML);
}
printList();
is this a good way of doing it ? thank you.
1 Answer
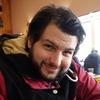
Eric M
11,546 PointsHi Anthony,
You've written a version of the printList()
function with no parameters, meaning that you can no longer pass it any data, it will simply run whatever you've written when called.
Something crucial you've left out of your snippet that explains why this works is the list definition at the top of the file.
I'm going to dive into a couple of code examples to hopefully show that while this works for this particular piece of example code Dave is working with in the video, it's generally not a good way to do things.
I'll be putting a lot of my post in the code comments so that it is right next to the thing I'm commenting on.
// this is the initalisation of the playList variable
// note that it is at the top of the file, not within
// any functions, this puts it in the global scope of
// this file - this means any functions will be able
// to call it directly
var playList =
[
'I Did It My Way',
'Respect',
'Imagine',
'Born to Run',
'Louie Louie',
'Maybellene'
];
// called by printList() for output, it will run
// document.write() on the argument passed to it
function print(message)
{
document.write(message);
}
// the printList function takes no parameters but
// instead accesses playList directly in its loop
function printList()
{
var listHTML = '<ol>';
for (var i = 0; i < playList.length; i ++)
{
listHTML += '<li>' + playList[i] + '</li>';
}
listHTML += '</ol>';
print(listHTML);
}
printList();
But what if I want to print more than one list?
// Global array playList
var playList =
[
'I Did It My Way',
'Respect',
'Imagine',
'Born to Run',
'Louie Louie',
'Maybellene'
];
// Global array vaporwaveList
var vaporwaveList =
[
'Outrun',
'Great Leader Has Fallen',
'Interceptor',
'Protovision',
'Current Events'
];
// the printList function takes no parameters but
// instead accesses playList directly in its loop
// there is no way to print the vaporwaveList!
function printList()
{
var listHTML = '<ol>';
for (var i = 0; i < playList.length; i ++)
{
listHTML += '<li>' + playList[i] + '</li>';
}
listHTML += '</ol>';
print(listHTML);
}
// to print the vaporwaveList we either need to
// rewrite printList() or create a new function
function printAnyList(theListToPrint)
{
var listHTML = '<ol>';
for (var i = 0; i < theListToPrint.length; i ++)
{
listHTML += '<li>' + theListToPrint[i] + '</li>';
}
listHTML += '</ol>';
print(listHTML);
}
// we'd probably delete printList() now, because printAnyList()
// does the same thing but we can use it for both lists!
// instead of typing two identical functions, we make one function
// work with multiple pieces of data
// why write printList(); printVaporwaveList(); when we can just:
printAnyList(playList);
printAnyList(vaporwaveList);
Well that's one perspective. What if we only want to print one list, but it's not in the global scope? The printList() function in your example only works because it knows what playList is - the array is in the global scope, so the function's inner code can access it.
As programs get larger we often have to deal with functions and data in multiple varying scopes, we can't just access anything from anywhere.
This below example will fail, because playList has been moved into another function's scope, our printList() function can't look inside that function's scope unless it is told explicitly to do so.
// the printList function looks for a playList variable
// in its scope and the global scope, but it doesn't
// find one, and won't be able to print our list
function printList()
{
var listHTML = '<ol>';
for (var i = 0; i < playList.length; i ++)
{
listHTML += '<li>' + playList[i] + '</li>';
}
listHTML += '</ol>';
print(listHTML);
}
// perhaps here there is a way for the user to make
// their own playlist, based on their input, so it
// is not hard coded into the global scope
function makePlayList()
{
// array scoped to the makePlayList() function
var playList =
[
'I Did It My Way',
'Respect',
'Imagine',
'Born to Run',
'Louie Louie',
'Maybellene'
];
printList();
}
However if printList() took any list as a parameter we could pass playList as an argument in our printList() call within makePlayList()
function printList(list)
{
var listHTML = '<ol>';
for (var i = 0; i < list.length; i ++)
{
listHTML += '<li>' + list[i] + '</li>';
}
listHTML += '</ol>';
print(listHTML);
}
function makePlayList()
{
// array scoped to the makePlayList() function
var playList =
[
'I Did It My Way',
'Respect',
'Imagine',
'Born to Run',
'Louie Louie',
'Maybellene'
];
// pass array to printList() function
printList(list);
}
I hope that helps you understand why we design functions around interacting with objects (groups of data) rather than one express thing. In the long run this style is easier to maintain and results in writing code that is smaller and more useful.
Cheers,
Eric
anthony amaro
8,686 Pointsanthony amaro
8,686 Pointsyes, i understand now. thank you so much for taking the time to explain it to me. functions are one of the hardest things for me to fully understand. but thanks to your example, it made it much clear for me. again thank you eric!.