Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial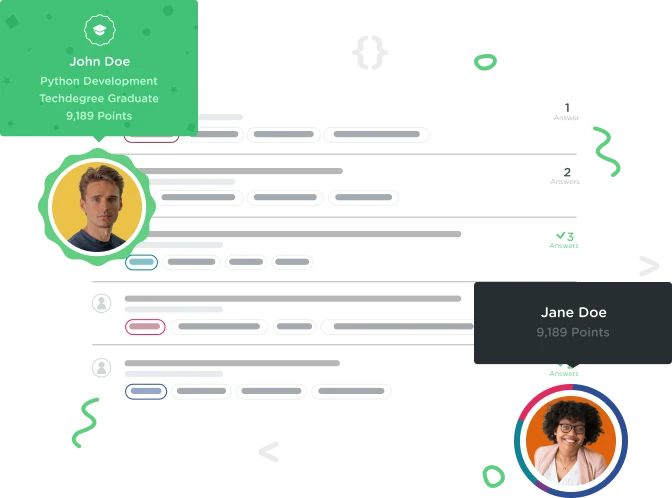

Jamil Jones
10,852 PointsWhat I'm a doing wrong here? This item will not pass.
I am following the direction exactly how it states. But it will not pass. What am I missing??
func coordinates(for location:String) -> (Double, Double) { }
func coordinates(for location:String) -> (Double, Double) {
}
1 Answer
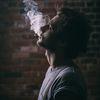
Lucian Rotari
12,007 PointsHey Jamil, there is nothing wrong with your code, you just didn't finish the challenge.
Let have a look, they ask you to declare a function and that's what you have done. What you missed is that your function MUST return a value.
func coordinates(for location:String) -> (Double, Double) {}
// -> (Double,Double) means that this function MUST return a tuple-type
Now the action is required here "For example, if I use your function and pass in the string "Eiffel Tower" as an argument, I should get (48.8582, 2.2945) as the value. If a string is passed in that doesnβt match the set above, return (0,0)"
So you have 3 locations
- Eiffel Tower
- Great Pyramid
- Sydney Opera House
Each of them has latitude and longitude:
- Eiffel Tower - lat: 48.8582, lon: 2.2945
- Great Pyramid - lat: 29.9792, lon: 31.1344
- Sydney Opera House - lat: 33.8587, lon: 151.2140
So your function must return the lat and lon for the location that user required.
For example if user required latitude and longitude for "Effel Tower" your function should return this values : (48.8582 , 2.2945)
In this case your function should look like that:
func coordinates(for location:String) -> (Double, Double) {
if location == "Eiffel Tower" {
retrun (48.8582 , 2.2945)
}else {
retunr (0,0)
}
}
But what if user has required another location? Then your function will return only (0,0). In this case you should have a solution for all 3 location and it's a good idea to use switch statement. And if you have learned about switch statements then your solution should look like that:
func coordinates(for location: String) -> (Double, Double) {
switch location {
case "Eiffel Tower" : return (48.8582 , 2.2945)
case "Great Pyramid" : return (29.9792 , 31.1344)
case "Sydney Opera House" : return (33.8587, 151.2140)
// But if user required an unknown location return a default value
default : return (0,0)
}
}