Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial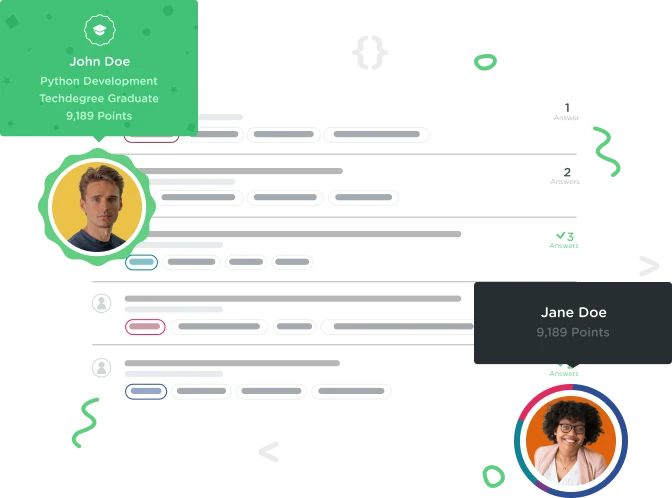

Hariidaran Tamilmaran
iOS Development Techdegree Student 19,305 PointsWhat is a Class?
Hello. I have no idea what a "class" in Java. Can someone please explain?
1 Answer
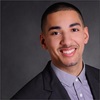
Mario Blokland
19,750 Pointsa class in Java is like a Blueprint of an object. A class describes how this object should behave and what for kind of attributes it has.
Let's say I want to create a class for a car. I know it has a name, a color, 3 or 5 doors and so on. These properties don't define the behavior of my car, thus you would add those as variables in the class and not as methods, for expample:
public class Car {
String color;
String name;
int doors;
}
The car can start, you should be able to break and so on. Those properties describe the behavior and would be added as methods to the class:
public class Car {
String color;
String name;
int doors;
public void startTheCar() {
// Do something
}
public void pressTheBreak() {
// Do something
}
}
Now you can take this class (the blueprint) and create a real object of it:
public static void main(String [] args) {
Car bmw = new car();
bmw.name = "bmw";
bmw.color = "black";
bmw.doors = 5;
bmw.start()
}
Hope that made it a little bit more clear for you. If you still have questions, please don't hesistate to ask mate.
Hariidaran Tamilmaran
iOS Development Techdegree Student 19,305 PointsHariidaran Tamilmaran
iOS Development Techdegree Student 19,305 PointsHow do we tell that there are many objects in a .java file?
Hariidaran Tamilmaran
iOS Development Techdegree Student 19,305 PointsHariidaran Tamilmaran
iOS Development Techdegree Student 19,305 PointsI also cannot understand what an "object" and a "method" are.
Jerry Kong
5,672 PointsJerry Kong
5,672 PointsHi Mario,
Is "object" always a variable? e.g. the number of doors(int), sun roof or not(Boolean), color of paint(String)
Is "method" always a function? e.g. do_something(), do_another_thing()
Edit: I think I'm incorrect. In the example: Car is the class. bmw is the object? name/color are instances variables?
name564681351614
3,497 Pointsname564681351614
3,497 PointsHow do we know that those properties "startTheCar()" and "pressThe Break()" relate back to the class "Car"?