Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial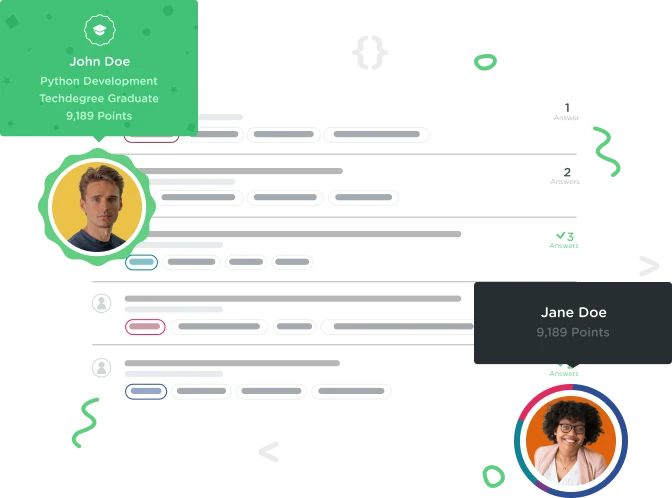

Craig Curtis
19,985 PointsWhat is a good use-case for the ES2015 Static Methods?
Furthermore, what would be certain use-cases for the 2 methods mentioned in the video?
// default static method
class Bird {
static changeColor(color) {
this.color = color;
}
constructor({ color = 'red' } = {}) {
this.color = color;
}
}
let redBird = new Bird;
Bird.changeColor.call(redBird, 'blue');
// Instance method
class Bird {
static changeColor(bird, color) {
bird.color = color;
}
constructor({ color = 'red' } = {}) {
this.color = color;
}
}
let redBird = new Bird;
Bird.changeColor(redBird, 'blue');
3 Answers

Tom Cluster
297 PointsIt is common to use static methods as an alternative constructor.
Moreover, they are frequently used in order to define utility and helper functions.
Take a look at this quick easy example:
class Bird {
constructor({ color = "red" } = {}) {
this.color = color;
}
static compareBirds(first, second) {
return (first.color === second.color);
}
}
let redBird = new Bird;
let blueBird = new Bird({"color": "blue"});
console.log(Bird.compareBirds(redBird, blueBird)); // false
console.log(Bird.compareBirds(redBird, redBird)); // true
As you can spot, static method compareBirds()
provides us with an ability to compare colors of already instantiated birds.
Of course it is a simplified example, but likewise, you can create a method for bird sorting. This method will also act as a helper function for your app.
Don't forget about new ...rest
parameter, which might be helpful in case you want to sort many birds! :)

Casey Ydenberg
15,622 PointsWhether or not there's EVER a use case for classes in ES6 is controversial ... https://medium.com/javascript-scene/common-misconceptions-about-inheritance-in-javascript-d5d9bab29b0a#.rgk7ty7ed Remember that class
is syntactic sugar - you're just creating an object (which can be used as a prototype for other objects).
The best use case for static methods in other languages is to group functions (that aren't really object-oriented and don't work on objects) together into classes for convenience and to create "alternate" constructors that are associated with the class their intended to construct. In JavaScript, you don't need any of this, but if you code in the classical pattern long enough you'll no doubt discover a use-case for them on your own (until then I wouldn't worry about it).
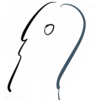
akak
29,445 PointsReact has a good use case of a static method.
Not going into details of how react works, a React Component uses PropTypes, an object that helps with validation of data coming into it. In ES6 you can declare it in two ways.
// adding it to the class outside of it
class SavedColors extends React.Component {
// react stuff
}
SavedColors.PropTypes = {
colors: React.PropTypes.objectOf(React.PropTypes.number).isRequired,
saved: React.PropTypes.bool.isRequired,
selectColor: React.PropTypes.func.isRequired,
isOpen: React.PropTypes.bool.isRequired
}
// adding it as a static method to the class
class SavedColors extends React.Component {
constructor() {
// react stuff
}
static PropTypes = {
colors: React.PropTypes.objectOf(React.PropTypes.number).isRequired,
saved: React.PropTypes.bool.isRequired,
selectColor: React.PropTypes.func.isRequired,
isOpen: React.PropTypes.bool.isRequired
};
// more react stuff
}
Basically you achieve the same thing but I think doing in the class itself is cleaner.

Lars Reimann
11,816 PointsYou cannot add static fields to a class this way (yet?). Instead you need to define a static getter like so:
static get PropTypes() {
return // stuff;
}