Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial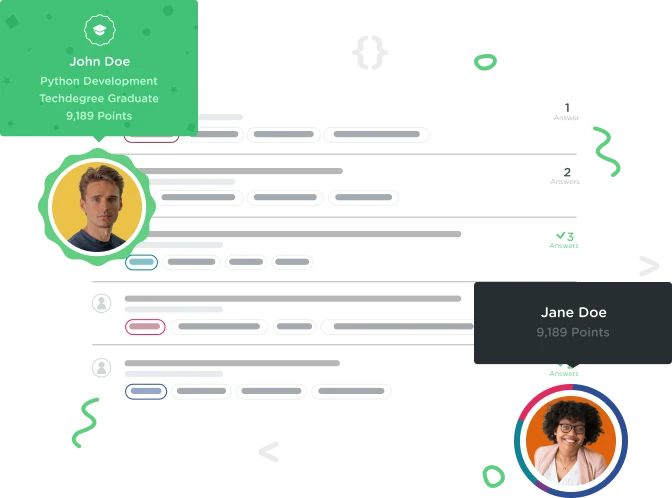
2 Answers
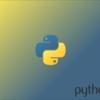
Kevin Faust
15,353 PointsHey Alexander,
First what's a class? a class is like a blueprint. You see how each file has that public class statement in the top? everything inside those class { } brackets is a blueprint.
Our blueprints cant come to life on their own, in order to do that we have to create an object. an object is basically the real life version of a blueprint. Consider we have something like public class robot followed by a bunch of code inside which is a blueprint for that robot. We have to create the object in our main file which turns it into a real robot. Lets take a look at our main file:
Game game = new Game();
Prompter prompter = new Prompter();
This is the general format of creating an object. We are basically taking our game/prompter blueprints, and summoning them to life. Now all the methods, instance variables, etc. inside that class is now ready to use under the object variable name. in our case it would be "game" and prompter". The first uppercases words such as "Game" and "Prompter" are the object types aka the blueprint class.
Ok now let's move onto the instance/member variables:
First off, member variables are a way of naming instance variables. member variables only exist under instance variables. So let's just look at this brief example from the code:
Lets look at the prompter and game files:
public class Prompter {
private Game mGame;
public class Game {
public static final int MAX_MISSES = 7;
private String mAnswer;
private String mHits;
private String mMisses;
See how we have some instance variables? these are the variables that belong directly to the object. we can use these anywhere in our class. Now, we also see how there is a little 'm' there right? that is not required for the code to work. it's pretty much just a naming convention. Whenever you want to make an instance variable, just make sure to put a little 'm' there. that way when we use that instance variable later on in our code, we can clearly see which is a instance and which is a local variable. When coming back to your code after let's say a few days, you can clearly see what type of variable it is. In general, it just makes your code cleaner and easier to read
I hope that cleared up your questions,
Happy coding and best of luck,
Kevin
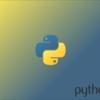
Kevin Faust
15,353 PointsHey Alex,
A 'member variable' is a variable that is defined inside an object and is accessible by all its methods. we have the 'm' prefix so we easily distinguish them. we can know which variables are local variables(variables that only exist inside a method) and which are instance variables(m/member variables) which are accessibly by all methods inside the class
Public class stuff {
String mTopic = "java"
public void sayHi () {
String hello = "whats up everyone";
System.out.println(hello);
}
public void favouriteTopic() {
System.out.println(mTopic);
}
}
Ok i put that thing above to illustrate. So we have a topic variable that belongs to the class. we can use that variable anywhere in our code. it belongs to the class/object. that's why we put the 'm' there so we can distinguish it from the second example. In our sayHi() method, it creates a string variable and then prints it out. we cannot use that variable anywhere else outside of that method. as you can see the 'm' is just a naming convention that we use to distinguish between the two types.
I hope that made sense,
Happy coding,
Kevin

Alexander Nowak
498 PointsAgh, so the member variable can be called on by any method in the class??
So is the class 'stuff' in the above code?
and what is a Class?
Why would you want to use a member variable over a instance variable?
Sorry, I know thats a lot of questions!
Thank you Kevin, I really appreciate your help!
Alexander Nowak
498 PointsAlexander Nowak
498 PointsThank you Kevin.
I really do appreciate the time you take to answer my questions so clearly.
Thanks
Alex
Kevin Faust
15,353 PointsKevin Faust
15,353 PointsGlad to have been of help :)