Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial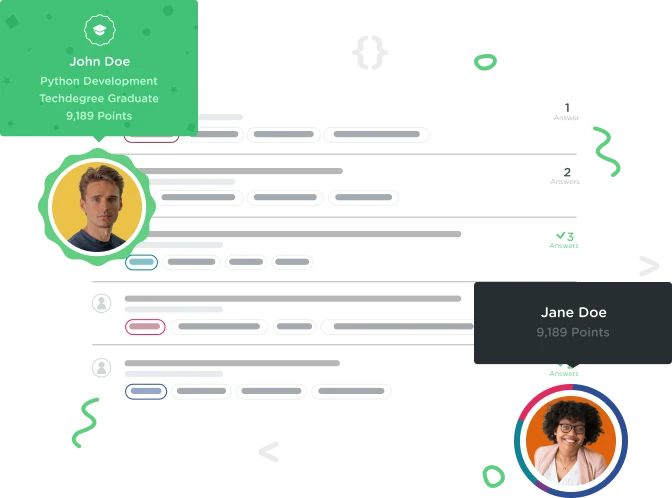
Miguel Nunez
3,266 PointsWhat is a PHP argument?
Please don't give me the main official or google links explaining what a php argument is because they explain it in a way that is very difficult to understand so can any one explain it what it means in simplicity and tell me what is it used for and why you should use it and what is it meant that they are like a variable which I still don't see how they are similar to a variable in comparison .
2 Answers

Christian Andersson
8,712 PointsDo you mean function parameters?
To understand the concept of function parameters, you'd have to understand what functions are and why/how they are used. So let me start with that.
A function is a block of code that is somewhat abstracted from the rest of your "typical" code. They are commonly used whenever you want to execute a routine procedure. Here's an example:
<?php
$a = 5.6;
$b = 3.1;
$foo = 4.9;
$bar = 18.7;
$result1 = doMagic($a, $b);
echo "The result of the first operation is: " . $result1;
function doMagic($var1, $var2) {
$sum = $var1 + $var2;
if($sum > 10) {
$sum = ceil($sum);
} else {
$sum = floor($sum);
}
return $sum;
}
$result2 = doMagic($foo, $bar);
echo "The result of the second operation is: " . $result2;
?>
In this example, we start off by declaring some variables. Then, we call the doMagic()
function and give it two argument parameters - the $a
and $b
variables. The code then "jumps" to the function part and executes it. The function can be declared anywhere in the code, and it can be called anywhere in the code as well. As you can see, I call the function twice, both before and after its' declaration. The code will "jump" to that part whenever called, and once finished it will resume executing on the line it had jumped from.
As I explained above, functions are abstract from the rest of the code. This means that within the function, you cannot use any of the $a
, $b
, $foo
or $bar
variables. It is isolated so it cannot "see" them. This might seem weird at first, but the purpose of a function is to carry out a routine - so it shouldn't be affected by the rest of the code.
However, sometimes (usually) we still want to give it some data to work with. Those are called the parameters of a function. In this example they are the $var1
and $var2
variables. These variables are local to the particular function. They only exist during the execution of the function and the rest of the code cannot see them.
So when you do doMagic($a, $b)
you tell the program to execute the function and you give the **value of $a to $var1 and the value of $b to $var2`. The function then does what it's supposed to do and returns the result.
Function arguments don't need to be a variable, they can be an explicit value too. For example: doMagic(1.2, 5.3)
.
Hope that clears things up for ya :)
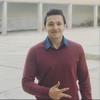
Hazem mosaad
15,384 Pointssimplest Explain Of Arguments ,,
//Now If You Want To Create A (process) Some Times for example :- you want to add two variables
$a = 5;
$b = 10;
// if you want to add them so you want create 3rd variable $sum to add them ,,
$sum = $a + $b;
// ok its easy but if you want to sum two number More Than One Time
$a = 5;
$b = 10;
$c = 4;
$d = 10;
// so you must create two sum variables to do this
$sum1= $a + $b;
$sum2 = $c + $d ;
//but if you create a function
function sum ($first_number_you_want_to_add_it,$second_number_you_want_to_add_it)
{
$sum = $first_number_you_want_to_add_it + $second_number_you_want_to_add_it;
return $sum;
}
//so if now we can do it easy
$c = sum($a,$b); where $a and $b two arguments must send to function sum to work
we do this process if we had block of code want to do it more time in diff. places like loop write one code
// and repeat it more time :)
Miguel Nunez
3,266 PointsMiguel Nunez
3,266 PointsSo $var1 and $var2 in your example are consider an argument? Sorry I kind of got lost I just want to make sure I understand and what is the purpose of an argument why would I use it or why would any one use it what's so special about it?
Christian Andersson
8,712 PointsChristian Andersson
8,712 PointsMiguel Nunez, the $var1 and $var2 would be the parameters of the function. The other variables $a, $b, etc are sent in as the arguments when you call the function. Quick explanation:
What's important here is to understand that a function is isolated, in a sense, from the rest of the code meaning that it cannot access variables as usual. For example trying to access $a inside the function wouldn't work.
The only way you can "give" the function certain data is by sending that data as arguments. The arguments is what's between the parenthesis in the function call, as explained in the example above.
So to answer your question "why would anyone use it" and "what is the purpose of an argument" - the answer is: to "send" data to the function. Remember what I said about isolation - if you couldn't send data to it, then it wouldn't be very useful.
Imagine you want to write a function that takes 2 numbers and returns the biggest one. The logic would be performed within the function block, but it wouldn't be a very useful function if you couldn't tell it **WHICH* numbers to compare. So you tell it by sending those, or "passing" them as it's called, as arguments to the function.
In the above example,
doMagic()
is called once and we pass the variables $a and $b to the function. When the function is run $var1 will have the value of 5 and $var2 will have the value of 8 - because that's what we passed in when we called it.So in a way, $a corresponds to $var1 and $b corresponds to $var2. So now you might wonder why we don't just use the same name for both - for example why write the code like this instead:
$a now corresponds to $a and $b to $b - makes more sense, right? While this is perfectly VALID, its probably not encouraged in most scenarios. Here's why:
Imagine you have the last code snippet, and you now decide to use this function to calculate more stuff:
See why it doesn't make much sense now? You can easily confuse what $a and $b are - are they the ones that were part of the main script or the ones used inside the function? (Recall what I said about isolation).
Hope this helps :)