Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial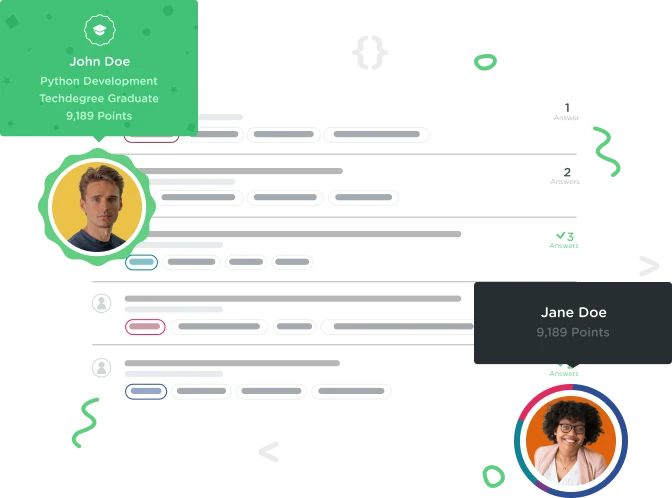
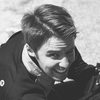
Brandon Leichty
Full Stack JavaScript Techdegree Graduate 35,193 PointsWhat is a Sequelize migration used for?
Hello Treehouse community,
I've been struggling with this for a few months now, and decided I'd finally ask the question in this forum.
I'm trying to understand what a migration is in Sequelize, and when/how it's used.
When Googling for my answer, I found a lot discussion on how to create a migration--but was unable to find the "why" behind what it is.
Any insight on this would be greatly appreciated. I'm trying to have a fundamental understand of what this is--so I can better understand how Sequelize works.
Thank you so much!
Brandon
PS: This is an example of the code I use to generate my Sequelize models (and migrations):
sequelize model:create --name Page --attributes "name:string, text:text, url:string"
1 Answer

Alexander La Bianca
15,959 PointsHi Brandon,
Good question. I can explain what I use it for in my personal project.
I use sequelize with MySQL as the database. When I first started the project I created all my sequelize models and defined what their relationships are to eachother. Like primary keys/foreign keys etc. Now I needed that model strucuture to also be reflected in my database tables. I could have manually created each table and create each column relationship in MySQL and there is no problem with that. However, you can create migration files that do that for you. So I can literally create a migration file along each model I created in the beginning and then run the migration command. That created all the tables for me and automatically set up the relations as well.
Now, lets say I add a new property to one of my models that I also need to store in the database. I can just add the property to my js model and create a migration file where I specify to add a new column for the model's table and what relationship it has.
The below is an example for creating a new table from one of my models. The migration is just an object literal with 2 function.s 'up' and 'down' up meaning what to do when running the migration. down meaning what to do when undoing your migration. Here I am simply creating a table on 'up' and dropping that very same table if I decide to undo the migration with 'down'.
module.exports = {
up: function (queryInterface, Sequelize) {
return queryInterface.createTable('product_orders',
{
id: {
type: Sequelize.INTEGER,
primaryKey: true,
autoIncrement: true
},
productId: {
type: Sequelize.INTEGER,
references: {
model: 'products',
key: 'id'
},
allowNull: false
},
orderId: {
type: Sequelize.INTEGER,
references: {
model: 'orders',
key: 'id'
},
allowNull: false
}
createdAt: Sequelize.DATE,
updatedAt: Sequelize.DATE,
}
)},
down: function (queryInterface, Sequelize) {
return queryInterface.dropTable('product_orders')
}
};
Now lets say you added a new property to your model and need that reflected in the db. Again, you see the up and down methods I can call. But instead of createTable or dropTable it is addColumn or removeColumn to account for the new property.
module.exports = {
up: (queryInterface, Sequelize) => {
return queryInterface.addColumn('product_orders','price', Sequelize.STRING)
},
down: (queryInterface, Sequelize) => {
return queryInterface.removeColumn('product_orders', 'price', Sequelize.STRING)
}
};