Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial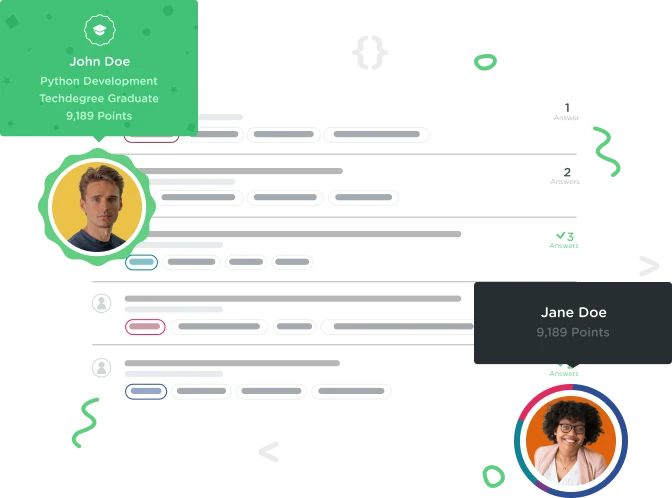

Matt Frerichs
4,015 PointsWhat is a Void return type?
I am having trouble understanding exactly what the void "()" return type does. I don't understand why you can't just use a return type of Int since the function parameter is of type Int.
2 Answers

Martin Wildfeuer
Courses Plus Student 11,071 PointsIf you do not want to return anything from your function, you use either -> Void
, -> ()
or nothing at all. Moreover, the return type has nothing to do with the parameters at all, you could easily return a String representation of a math operation that requires two Ints.
// We just want to print to the console,
// so we don't need to return anything
func sayHello() {
println("Hello")
}
// You would call this function
// without assigning it to a variable/constant
sayHello();
// That would trigger a warning, as
// the function returns nothing
let test = sayHello()
// Oh, and that would trigger an error, as we
// don't expect the function to return anything...
func sayHello() {
return "Hello"
}
// ...whereas this also triggers an error. If we specify
// a return type, we have to return something
func sayHello() -> String {
}
Why would we need a function that returns nothing?
That is a very common use case. For example, if you had a custom view that you wanted to hide, you would implement that method in the class so you can call it from outside.
class CustomAlert : UIViewController {
...
func show() {
// Code to show this view
}
func hide() {
// Code to hide this view
}
}
let customAlert = CustomAlert()
customAlert.show()
See? There is no return value needed at all!

Greg Kaleka
39,021 PointsHi Matt,
The concept Pasan Premaratne is going over is pretty complicated, but to answer your question specifically, a function that returns void is essentially one that doesn't return anything.
This function has a return type string, so I can pass its returned value to a constant:
func sayHello(name: String) -> String {
return "Hello, \(name)"
}
let greeting = sayHello("Matt") // I can do this because sayHello spits out a String
This function has a return type of void, so it doesn't spit anything out that I can use:
func logError(name: String) -> () { // the arrow and parens are entirely optional
println(\(name) did something wrong!) // sorry, couldn't resist :)
}
Does that make sense?
In Pasan's example, that inner function is just printing something to the console, rather than returning a value. Functions with void as a return type are pretty common - they are handy for doing "work" but not necessarily returning something you would use elsewhere. The function viewDidLoad is probably the best example of a function with a return type of void.

Matt Frerichs
4,015 PointsThank you for the help!

Greg Kaleka
39,021 PointsSure thing!
Matt Frerichs
4,015 PointsMatt Frerichs
4,015 PointsThis helps clear things up for me. Thank you for the help!
Martin Wildfeuer
Courses Plus Student 11,071 PointsMartin Wildfeuer
Courses Plus Student 11,071 PointsYou are welcome!