Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial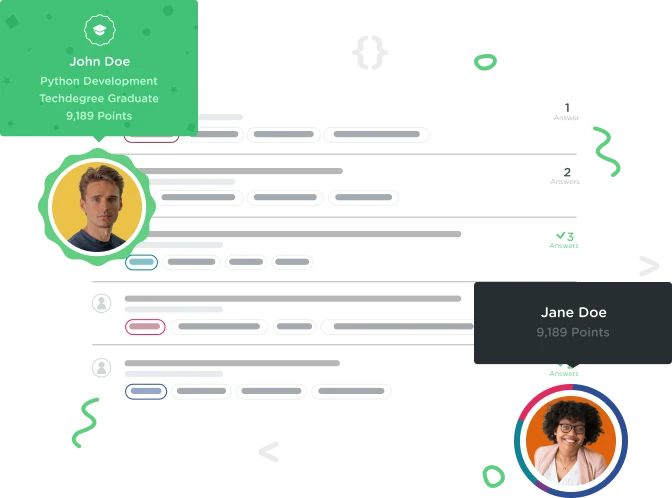

Sahil Gangele
6,851 PointsWhat is an instance variable in objective c?
Could you give me an in-depth explanation with examples.
Thank you so much
1 Answer
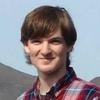
nicktroccoli
3,765 PointsInstance variables are basically pieces of information specific to an individual instance of a class. For example, if we have a class called Car, an instance variable on car might be the number of miles on a car - that number is specific to an individual car. In Objective-C, instance variables are commonly created with @propertys. An @property is basically an instance variable with a few extra bonus features attached. The biggest addition is that Objective-C will automatically define what's called a setter and a getter for you automatically. What that basically means is it makes two methods for you automatically that let you set and get the value of the instance variable, respectively.
@propertys are created inside the @interface section of either your .h or .m files (which makes the @property public or private, respectively). The way you create a new @property is by saying
@property(nonatomic, strong) UIImage *myImage;
This example will create a new instance variable of type UIImage called myImage. It also adds two new methods to your class: [-myImage], the getter, and [-setMyImage:], the setter, which takes a UIImage as a parameter and changes myImage to be that image. Lets do another example - our Car class:
@property (nonatomic, strong) NSString *carModel;
@property (nonatomic, strong) NSNumber *milesDriven;
@property (nonatomic, strong) NSNumber *numberOfDoors;
This defines 3 new instance variables - a string for the car's model (e.g. "Mustang") a number for the number of miles on the car, and a number for the number of doors the car has. The format for declaring an @property has a couple of parts. After @property, you can put different values inside the parentheses to specify certain "extras" that the @property can come with. For example, if you only want a getter, but not a setter (to prohibit setting the value of an instance variable publicly) you can add readonly inside parentheses. Another attribute, used in the example above, is the nonatomic attribute. Nonatomic means the @property isn't thread-safe, (you'll almost always use nonatomic in @propertys). Strong is another attribute; the strong attribute means that this property is "owned" by this object (the alternative to the strong attribute is the weak attribute - for more info on strong vs. weak take a look at this post which does a great job at explaining properties and the different attributes you can add in parentheses). After the attributes you put the type of the instance variable, and finally you put the name. Hope this gives you a better idea of how @propertys work!