Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial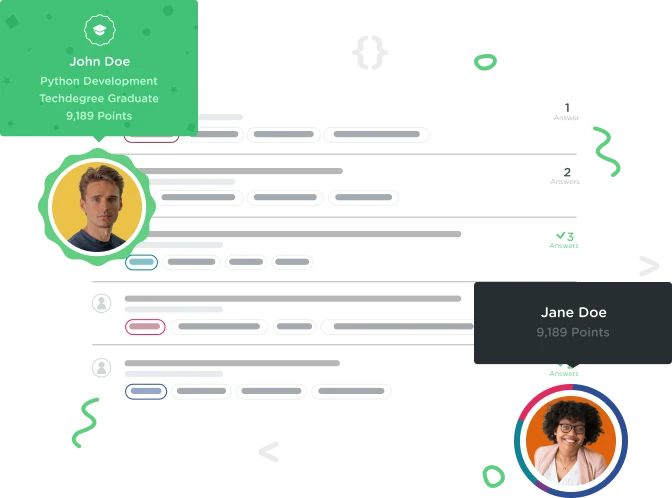

JJ Brandler
988 Pointswhat is being compared in "if(equals(other))"
when we call the compareTo method, we use the mDescription and mCreationDate. but when we call the equals method, what is it being compared to? (from the Java Data Structures, Interfaces)
4 Answers
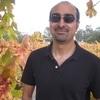
Kourosh Raeen
23,733 PointsThe equals method is called on the current object. So this code:
if (equals(other)) {
return 0;
}
is equivalent to this code:
if (this.equals(other)) {
return 0;
}
In the first version the this
keyword is omitted.
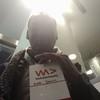
Dennis Addo
Courses Plus Student 2,943 Pointscan you post in an example so that we can help you solve the problem or do you want a general understanding of the CompareTo Interface?

JJ Brandler
988 PointsI am trying to get better understanding of what is going on.
here is the code:
@Override
public int compareTo(Object obj){
Treet other = (Treet)obj;
if(this.equals(other))
return 0;
int dateComp = mCreationDate.compareTo(other.mCreationDate);
if (dateComp==0)
return mDescription.compareTo(other.mDescription);
return dateComp;
}
I found that the code still works if i use
if(this.equals(other))
in place of if(equals(other))
I found the use of if(equals(other)) hard to understand, and I am trying to wrap my head around how it is being used in this case, and when I can/should use it like that.
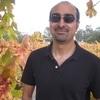
Kourosh Raeen
23,733 PointsHi JJ - See my answer above.

JJ Brandler
988 PointsKourosh Raeen, when would you "this" and when would you leave it out?
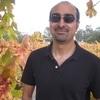
Kourosh Raeen
23,733 PointsIf there is any ambiguity then you need to use this
. As an example, consider the constructor in the Treet class:
public Treet(String author, String description, Date creationDate) {
mAuthor = author;
mDescription = description;
mCreationDate = creationDate;
}
Now, suppose that when defining the member variables we hadn't started the variable names with m:
private String author;
private String description;
private Date creationDate;
Then suppose you write the constructor code like this:
public Treet(String author, String description, Date creationDate) {
author = author;
description = description;
creationDate = creationDate;
}
This code is now ambiguous in the sense that when you say author = author it is not clear that which one is the member variable and which one is the parameter. So to fix it we use the this
keyword:
public Treet(String author, String description, Date creationDate) {
this.author = author;
this.description = description;
this.creationDate = creationDate;
}