Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial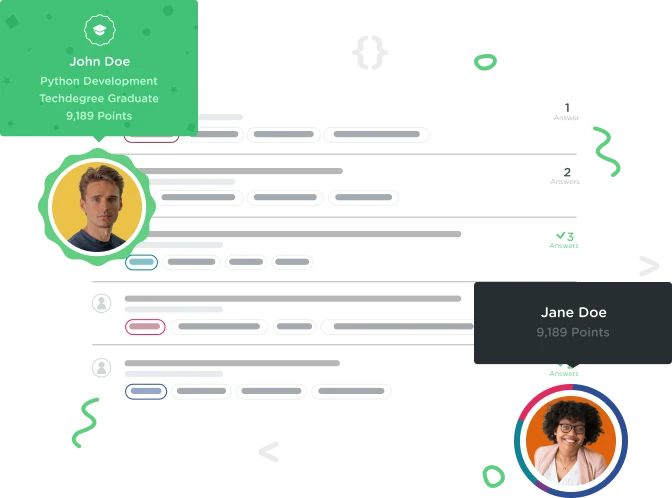

pratyush raj
7,097 Pointswhat is casting and override???
i have two questions:- 1.what is casting please explain with an example?? 2.what is override????
1 Answer

Aaron Bowes
4,465 PointsType casting is a way to tell the compiler to treat an instance of an object as a more specific type of object further down the family tree. That's a mouthful so let me break it down by building up to it with the example used in the videos.
If you remember, we first created an Animal class. This animal class has a property called sound and a method called makeSound. In code, it looks like this:
class Animal {
String sound = "";
void makeSound() {
System.out.println(sound);
}
}
Next, we created another class called Dog. We also said that the Dog class would inherit from the Animal class. At this point, without putting anything into the Dog class, the Dog class will behave exactly like the Animal class. It has the sound property and the makeSound method which simply prints out whatever is stored in sound. But we all know that a dog says "Bark" so we'll create a constructor that will set the sound string variable to "Bark".
class Dog extends Animal {
public Dog() {
sound = "Bark";
}
}
In other words, a Dog is a descendant of an Animal. If this were expressed as a family tree, it'd look like this: Animal -> Dog Where the Animal class is the parent of the Dog class.
In the videos, Ben created another class called DogFood which didn't extend anything and didn't have any properties or methods. It's a completely separate object.
class DogFood {}
In his example, he created an array to act as a list of all the objects you would need to give someone if they were watching your dog. This list would have the dog and the dog food. However, remember that in order to store items in an array, all the items have to be the same type. A Dog object and Dog Food object are completely different things. So, in order to store these items together in an array, we'll have to create the array with a type that these two items have in common. It turns out that everything extends ultimately from the Object class in Java. So, we can store these in an Object Array:
Object[] dogItems = {new Dog(), new DogFood()};
This is great until we want to pull that Dog object out of the list and call the makeSound method. This is where type casting comes in. In other words, this will not work:
dogItems[0].makesound();
If we wanted to call the makeSound method, we couldn't just pull it out of the list and call makeSound because at this moment, the Dog is only being seen as a basic Object, not as a Dog. Remember that the overall family tree looks like this for Dog objects:
Object -> Animal -> Dog
The base Object class doesn't have a method called makeSound. That method is further down the family tree (beginning in the Animal class). So, we do what's called type casting to tell the compiler "don't treat this as a basic Object here, treat it like something else". This is done before the object you want to cast and in parentheses:
((Animal) dogItems[0]).makeSound();
Here, I'm telling the compiler to treat dogItems[0] (the Dog) like an Animal since this is where the makeSound method is. I could have cast is as a Dog instead. But in this case, it doesn't matter since the Dog class's makeSound method isn't any different than the Animal class's.
To answer your second question, overriding a method is simply changing what a method does compared to the base (also called super) class. There's usually an "Override" annotation above the method you're overriding. Here's an example.
Let's say you want the Dog class to do everything that the Animal class does but you want to change what it does when makeSound is called. You would do it like this:
class Dog extends Animal {
// This is the annotation
@Override
public void makeSound() {
// Write whatever you want makeSound to do instead here
}
}
If you only want to add functionality to the makeSound method, call the "super" class and then add your functionality. Here's an example taken from the videos:
class Dog extends Animal {
@Override
public void makeSound() {
// Calling super which will get all of the original functionality from the Animal class
super.makeSound()
// New functionality - in this case, wags its tail
System.out.println("*Wags tail*");
}
}
I hope this answers everything for you! Rewatch "Casting Instances" and "Super Override" again if needed.
pratyush raj
7,097 Pointspratyush raj
7,097 PointsThanks it was really helpful u explained the concepts very well.
Aaron Bowes
4,465 PointsAaron Bowes
4,465 PointsThank you and you're welcome! I'm glad it helped!
Steven Yang
7,515 PointsSteven Yang
7,515 PointsThank u so much. This is everthing i want to know!!!
CamilleMarie Paugam
1,521 PointsCamilleMarie Paugam
1,521 PointsThis is the most amazing explanation I have ever seen!!! Thank you! I am a visual learner, but I also need a lot of description to understand. This really helped a lot. Thanks again!