Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial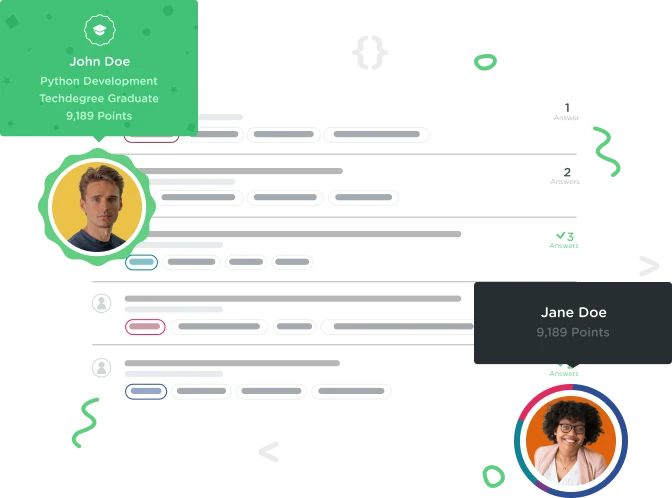

Susan Rusie
10,671 PointsWhat is causing these errors?
I am getting these errors in the console and I don't know why: One is saying that the controller is not defined in the todo.bundle .js and the main ctrl is not a function, not defined in the vendor. bundle.js file. What do you think could be causing this? Here is my code:
'use strict';
var angular = require('angular');
angular.module('todoListApp')
.service('dataService', function($http, $q){
this.getTodos = function(cb) {
$http.get('/api/todos').then(cb);
};
this.deleteTodo = function(todo) {
console.log("I deleted the " + todo.name + " todo!");
};
this.saveTodos = function(todos){
var queue = [];
todos.forEach(function(todo){
var request;
if(!todo._id){
request = $http.post('/api/todos', todo)
} else {
request = $http.put('/api/todos/' + todo._id , todo).then(function(result){
todo = result.data.todo;
return todo;
})
};
queue.push(request);
});
return $q.all(queue).then(function(results){
console.log("I saved " + todos.length + " todos!");
});
};
});
module.exports = DataService;
'use strict';
var angular = require('angular');
angular.module('todoListApp');
.controller('mainCtrl', function($scope, dataService){
dataService.getTodos(function(response){
var todos = response.data.todos;
$scope.todos = todos;
});
$scope.addTodo = function() {
$scope.todos.unshift({name: "This is a new todo.",
completed: false});
};
})
module.exports = MainCtrl;
'use strict';
angular.module('todoListApp')
.controller('todoCtrl', function($scope, dataService){
$scope.deleteTodo = function(todo, index) {
$scope.todos.splice(index, 1);
dataService.deleteTodo(todo);
};
$scope.saveTodos = function() {
var filteredTodos = $scope.todos.filter(function(todo){
if(todo.edited) {
return todo
};
})
dataService.saveTodos(filteredTodos).finally($scope.resetTodoState());
};
$scope.resetTodoState = function(){
$scope.todos.forEach(function(todo){
todo.edited = false;
});
};
});
module.exports = TodoCtrl;
Now, I am getting this error. I corrected everything else so this is what my code looks like now for my main.js :
'use strict';
function MainCtrl ($scope, dataService) {
dataService.getTodos(function(response){
var todos = response.data.todos;
$scope.todos = todos;
});
$scope.addTodo = function() {
$scope.todos.unshift({name: "This is a new todo.",
completed: false});
};
}
module.exports = MainCtrl;
While Nodemon, Webpack, and Mongo are functioning as they should, I am still getting this error when I run it in the developer tools:
todo.bundle.js:73 Uncaught ReferenceError: DataService is not defined(…)(anonymous function) @ todo.bundle.js:73__webpack_require__ @ vendor.bundle.js:51(anonymous function) @ todo.bundle.js:31__webpack_require__ @ vendor.bundle.js:51(anonymous function) @ todo.bundle.js:11__webpack_require__ @ vendor.bundle.js:51webpackJsonpCallback @ vendor.bundle.js:22(anonymous function) @ todo.bundle.js:1
vendor.bundle.js:14035 Error: [ng:areq] Argument 'mainCtrl' is not a function, got undefined
http://errors.angularjs.org/1.5.8/ng/areq?p0=mainCtrl&p1=not%20a%20function%2C%20got%20undefined
at vendor.bundle.js:183
at assertArg (vendor.bundle.js:2007)
at assertArgFn (vendor.bundle.js:2017)
at $controller (vendor.bundle.js:10445)
at setupControllers (vendor.bundle.js:9566)
at nodeLinkFn (vendor.bundle.js:9347)
at compositeLinkFn (vendor.bundle.js:8735)
at compositeLinkFn (vendor.bundle.js:8738)
at publicLinkFn (vendor.bundle.js:8615)
at vendor.bundle.js:1878(anonymous function) @ vendor.bundle.js:14035(anonymous function) @ vendor.bundle.js:10582$apply @ vendor.bundle.js:17902bootstrapApply @ vendor.bundle.js:1876invoke @ vendor.bundle.js:4833doBootstrap @ vendor.bundle.js:1874bootstrap @ vendor.bundle.js:1894angularInit @ vendor.bundle.js:1779(anonymous function) @ vendor.bundle.js:31878trigger @ vendor.bundle.js:3322defaultHandlerWrapper @ vendor.bundle.js:3612eventHandler @ vendor.bundle.js:3600
alex novickis
34,894 Pointsalex novickis
34,894 Pointsin your mainCtrl
you have a line like this
angular.module('todoListApp'); .controller(....)
note the ;