Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial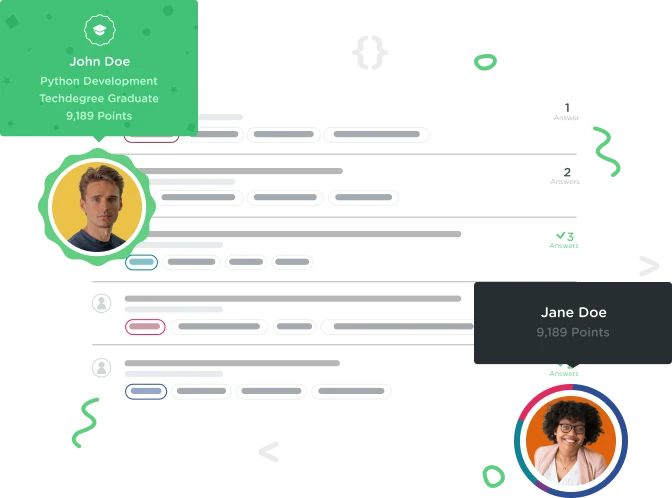

ammarkhan
Front End Web Development Techdegree Student 21,661 PointsWhat is constructor
I am finding difficult to understand constructor, I know it look like a method but when we can
constructor(){
pet(name){
this.name = name;
}
}
where does this.name comes from? As we haven't defined any property with that name? How is constructor different than a function?
4 Answers
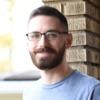
Justin Cantley
18,068 PointsJust like Ashley said in the video, if the class is the blueprint, the constructor is the factory that uses the blueprint to make an instance of the class. As an example:
class animal {
constructor(animal) {
this.animal = animal;
}
}
I can create instances of the class like so:
const spike = new animal('dog');
const spencer = new animal('cat');
This will create different instances of the animal class assigning a different value to each instance's this.animal property.
The 2 instance examples would be represented something like this:
spike {
this.animal = 'dog';
}
spencer {
this.animal = 'cat';
}

Kylie Soderblom
3,890 PointsStep 1: Create a new class class Pet {} //capital P is convention. Pet is the name of your newly created class. Step 2: include a constructor method. // This is your empty blueprint that will list the properties in your new class. class Pet { constructor ( empty ) { // no parameters yet
} Step 3: Add the common property names for the class. You will use "this." because it is referring to the new object under construction. Nothing is being constructed at this point. This is just the plan/blueprint. When they push the button - this is the recipe to make the following. Create a property (ex: animal) to this 'new' object under construction with the value of the given parameter.
class Pet { constructor (animal, age, breed, anythingYouChoose){ this.animal = animal; this.age = age; this.breed = breed; this.anythingYouChoose = anythingYouChoose; } } I know this answer came 2.5 years later but maybe it will help someone.
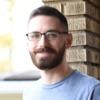
Justin Cantley
18,068 PointsYou don't have to define this.laptop or the other this.variables outside of the constructor in standard js.

ammarkhan
Front End Web Development Techdegree Student 21,661 PointsR u saying that my pasted code is not right?
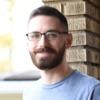
Justin Cantley
18,068 PointsCorrect. It isn't necessary to declare the 'this' variables outside of the constructor. And even if it was, you've declared them incorrectly. You must use a keyword like var, let or const before each variable declaration. For example:
var laptop;
let tablet;
const mobile;
ammarkhan
Front End Web Development Techdegree Student 21,661 Pointsammarkhan
Front End Web Development Techdegree Student 21,661 PointsThe way I know it works is like this
This is how I would do in Angular's TypeScript File. So my question was where are we defining the variable, that refer to this?