Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial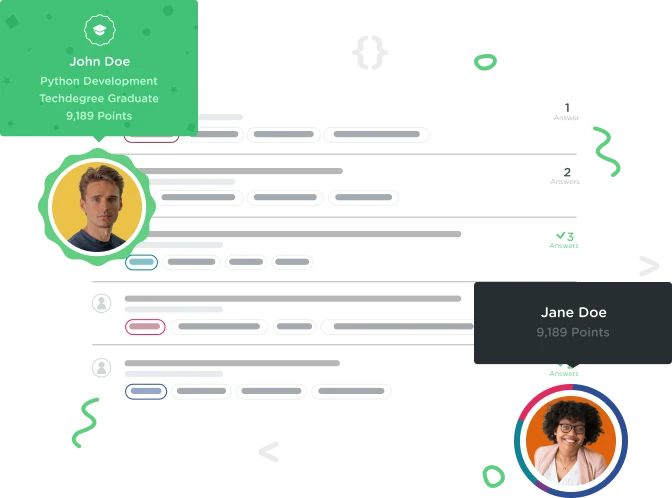

AbduRahman Ezzeldin
2,365 Pointswhat is done wrong here ?
i keep getting a compiler message of an unexpected symbol and it happens to be the one where i inherit the base value of a method the symbol is the " : " symbol and even if i dont get the base for the method entirely it still makes a compiler error ?
namespace Treehouse.CodeChallenges
{
public class SequenceDetector
{
public virtual bool Scan(int[] sequence)
{
return true;
}
}
}
namespace Treehouse.CodeChallenges
{
class RepeatDetector : SequenceDetector
{
private int _first;
private int _second;
public override bool Scan(int[] sequence) : base(sequence)
{
return !(sequence[_first] = sequence[_second]) ;
}
}
}
1 Answer
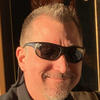
Peter Vann
36,425 PointsHi
You don't need:
: base(sequence)
You need to iterate over the elements in the sequence and do systematic comparisons of the current element and the preceding one, and if they are the same return true.
You need this in RepeatDetorctor,cs:
namespace Treehouse.CodeChallenges
{
class RepeatDetector : SequenceDetector
{
public override bool Scan(int[] sequence)
{
for(int i=1; i < sequence.Length ; i++)
{
if(sequence[i] == sequence[i - 1])
{
return true;
}
}
return false;
}
}
}
Keep in mind, with this logic it returns true when the first, if any, repeat is detected, which is what the challenge is asking for.
I hope that helps.
Stay safe and happy coding!