Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial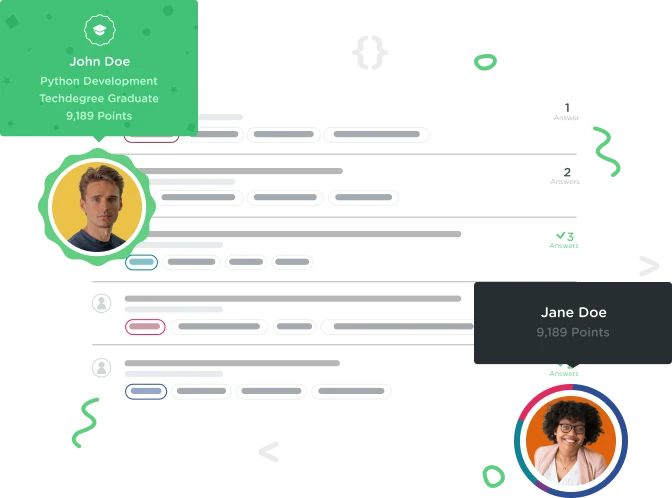
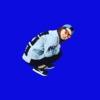
Lucy Jeong
7,243 Pointswhat is error 'Extra argument in call' ?
I have error about this code ForecastService.getForecast(coordinate.lat, long: coordinate.long)
the error is Extra argument in call
this code in following code
override func viewDidLoad() {
super.viewDidLoad()
let forecastService = ForecastService(APIKey: forecastAPIKey)
ForecastService.getForecast(coordinate.lat, long: coordinate.long)
{
(let currently) in
if let currentWeather = currently {
//update UI
}
}
}
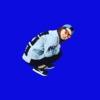
Lucy Jeong
7,243 PointsThank u,Martin this is ViewController import UIKit import Foundation
class ViewController: UIViewController {
@IBOutlet weak var currentTemperatureLabel: UILabel?
@IBOutlet weak var currentHumidityLabel: UILabel?
@IBOutlet weak var currentPrecipitationLabel: UILabel!
@IBOutlet weak var currentWeatherIcon: UIImageView?
@IBOutlet weak var currentWeatherSummary: UILabel?
private let forecastAPIKey = "0425b6e7c578ae4dcca0d30165c51266"
let coordinate: (lat: Double, long:Double) = (37.8267,-122.423)
override func viewDidLoad() {
super.viewDidLoad()
let forecastService = ForecastService(APIKey: forecastAPIKey)
ForecastService.getForecast(coordinate.lat, long:coordinate.long) {
(let currently) in
if let currentWeather = currently {
//update UI
dispatch_async(dispatch_get_main_queue())
{
//excute closure
if let temperature = currentWeather.temperature {
self.currentTemperatureLabel?.text = "\(temperature)°"
}
if let humidity = currentWeather.humidity{
self.currentHumidityLabel?.text = "\(humidity)%"
}
if let percipitation = currentWeather.precipProbability{
self.currentPrecipitationLabel?.text = "\(precipitation)"
}
if let icon = currentWeatherIcon.icon{
self.currentWeatherIcon?.image = icon
}
if let summary = currentWeather.summary{
self.currentWeatherSummary?.text = summary
}
}
}
}
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
}
and then, ForecastService is
import Foundation
struct ForecastService {
let forecastAPIKey: String
let forecastBaseURL: NSURL?
init(APIKey:String){
self.forecastAPIKey = APIKey
forecastBaseURL = NSURL(string: "https://api.forcast.io/forecase/\(forecastAPIKey)")
}
func getForecast(lat: Double, long: Double, completion:(CurrentWeather? -> Void)){
if let forecaseURL = NSURL(string: "\(lat),\(long)", relativeToURL: forecastBaseURL){
let networkOperation = NetworkOperation(url: forecaseURL)
networkOperation.downloadJSONFromURL {
(let JSONDictionary) in
let currentWeather = self.currentWeatherFromJSON(JSONDictionary)
completion(currentWeather)
}
} else {
print("Could not construct a valid URL")
}
}
func currentWeatherFromJSON(jsonDictionary: [String: AnyObject]?) -> CurrentWeather?{
if let currentWeatherDictionary = jsonDictionary?["currently"] as? [String: AnyObject]{
return CurrentWeather(weatherDictionary: currentWeatherDictionary)
} else {
print("JSON dictionary returned nil for 'currently' key")
return nil
}
}
}
Please, fix this error..:(
3 Answers

Aubrey Taylor
2,991 PointsI ran into the same error for a different reason. But I did solve it by making sure all my syntax was correct. In your case, if you look at your code you've capitalized your reference to ForecastService.
Change this:
let forecastService = ForecastService(APIKey: forecastAPIKey)
ForecastService.getForecast(coordinate.lat, long: coordinate.long)
To This:
let forecastService = ForecastService(APIKey: forecastAPIKey)
forecastService.getForecast(coordinate.lat, long: coordinate.long)
Hope that helps!
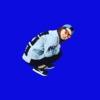
Lucy Jeong
7,243 Pointsthank you ! I fixed it! :)

Martin Wildfeuer
Courses Plus Student 11,071 PointsOk, it seems you are using the latest Xcode and Swift 2.X. This course was designed for Swift 1.2, you have already changed println to print, but error handling has also changed with Swift 2.X.
Accordingly, in the NetworkOperation class, serialization does not return a pointer to an error anymore, but utilizes the new Error handling.
You have to change this:
// 2. Create JSON object with data
let jsonDictionary = NSJSONSerialization.JSONObjectWithData(data, options: nil, error: nil) as? [String: AnyObject]
completion(jsonDictionary)
to this:
// 2. Create JSON object with data
let jsonDictionary = (try? NSJSONSerialization.JSONObjectWithData(data!, options: [])) as? [String: AnyObject]
completion(jsonDictionary)
// Please note that I am force unwrapping the `data` object,
// you might want to make sure it's not nil in advance.
See? NSJSONSerialization.JSONObjectWithData
does not have a third argument anymore. It used to be error
, but it has been omitted in favor of the new error handling, thus your error Extra argument in call.
Hope that helps!
P.S.
Xcode can also take care of converting to the latest swift syntax. If it does not ask you on project launch, you can also start conversion manually: Edit > Convert > To latest Swift Syntax

Aubrey Taylor
2,991 PointsApologies, I goofed on the markdown syntax:
Change this:
let forecastService = ForecastService(APIKey: forecastAPIKey)
ForecastService.getForecast(coordinate.lat, long: coordinate.long)
let forecastService = ForecastService(APIKey: forecastAPIKey)
forecastService.getForecast(coordinate.lat, long: coordinate.long)
Martin Wildfeuer
Courses Plus Student 11,071 PointsMartin Wildfeuer
Courses Plus Student 11,071 PointsThis looks right to me. Please post your entire ViewController and ForecastService class here, let's see if we can find something. Cheers!