Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial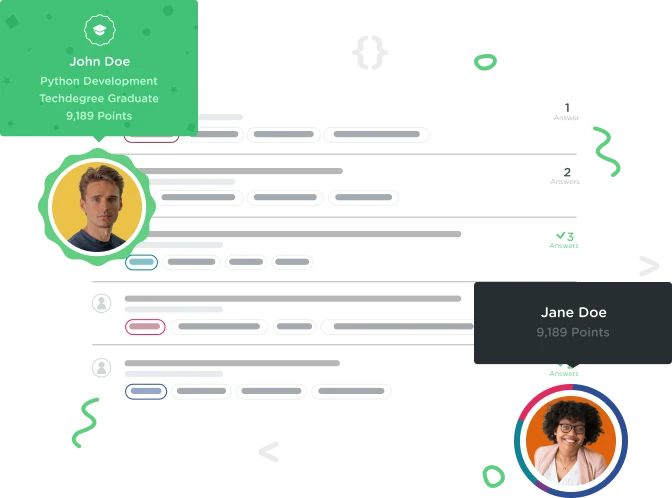
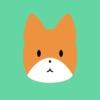
Mitul Patel
24,890 PointsWhat is Full Stack Javascript?
The title pretty much says it all. There has been a lot of talk about javascript in recent years. Javascript as a language has become very robust that it can now be used as server side language. But what makes a javascript developer a full stack developer with just javascript?
5 Answers

Aaron Graham
18,033 PointsOf the languages you mentioned, I have the most experience with PHP on Apache. Let me try and give you an answer in relation to that. With PHP on Apache, your browser makes an HTTP request to an Apache server. Let's say, http://example.com/index.php. The Apache server at example.com looks at the request, locates the resource, in this case index.php, parses the file, interprets any <?php ?>
scripts it finds, and sends the result back to your browser. With server side javascript, you use javascript to create the server. Your browser makes a request to the javascript server, the server processes the request using the javascript code you programmed it with, and the results are sent back to your browser.
For example, here is a quick and dirty http nodejs server:
var http = require('http')
, html = '<h1>Hello World</h1>';
http.createServer(function (req, res) {
res.writeHead(200, {'Content-Type': 'text/html'});
res.end(html);
}).listen(3000);
If this were saved in a file called app.js, you would run this using node like so: node ./app.js
. This server will listen on TCP port 3000, not the typical port 80, and doesn't really serve a valid HTML page, but hopefully you get the idea. Something similar in PHP, once you set up your Apache server, would look something like this:
<?php echo "<h1>Hello World</h1>"; ?>
Hopefully that gives you some idea as to the differences. There are other differences in how the servers themselves operate. For instance, Apache usually uses separate process to serve multiple requests. Each process will block incoming connections until it is finishes serving the request it is currently working on. Node uses a non-blocking event loop. Instead of blocking requests until it is finished serving the current request, the event loop sends the request to your code with a callback function to execute when any processing is done. Each has their strengths and weaknesses, and in the end you should probably learn a few different languages and the best use cases for each. Keep your toolbox full. If all you have is a hammer, everything looks like a nail.
Hope that makes sense.
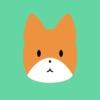
Mitul Patel
24,890 Points
Aaron Graham
18,033 PointsYeah. What he said. Good find! Incidentally, learn Node. It's awesome. It is incredibly flexible. A web server is just one of the many things you can build with it.

Aaron Graham
18,033 PointsFull stack javascript would be just what it sounds like; using javascript to code both the front end (browser) and the back end (server). The front end would usually include things like jQuery and Backbonejs, while the back end would be, more than likely, Nodejs. With other technologies, you need to know javascript for the browser, and something else, Ruby on Rails, PHP, etc. for the server side.

James Barnett
39,199 PointsNon-existent as it doesn't account for the OS, networking, caching, database.

Aaron Graham
18,033 PointsFair point. I guess it depends on how deep your definition of "full stack" goes. For the record, I tend to agree with you, but it is also accepted to mean, especially when talking about "full stack web development" or a "web stack", to mean just the browser, the server, and the database. I guess I left MongoDB out of my answer, but with that, you can pretty much manage the web stack with mostly javascript.
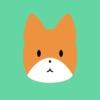
Mitul Patel
24,890 PointsI understand javascript as a front end language, but how does Javascript work as a server side language in comparison with languages like Ruby, PHP, and Python.