Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial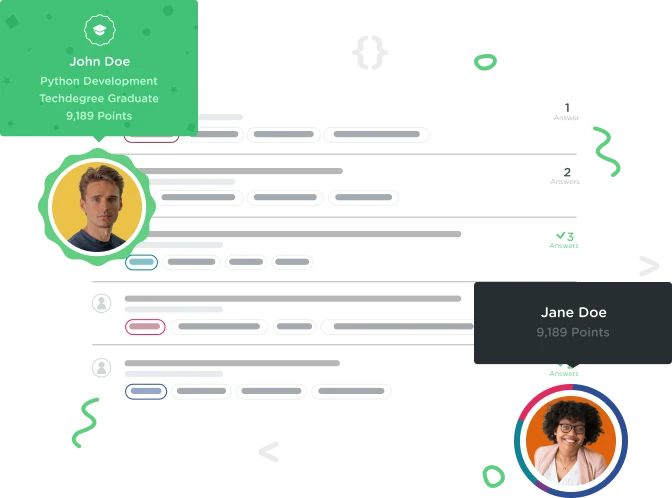

Farid Lavizadeh
12,006 PointsWhat is getJSON in getProfile returning to?
In the bellow function, there are 2 returns. I understand the "return profiles". I don't understand why there is an inner return for getJSON function. Where is it being passed to? And why wasn't it there before when the entire function was just a call back? (again I am referring to only to the first return. Does resolve(data) not return data?
function getProfiles(json) {
const profiles = json.people.map( person => {
return getJSON(wikiUrl + person.name);
});
return profiles;
}
Mod Edit: Fixed code formatting. See the Treehouse Markdown Guide for more information on how to do this.
2 Answers

JASON LEE
17,352 PointsThis answer is a bit late, so hoping it will help someone else that is having the same question (like I did).
From playing around with the code on a mock up version, I can try to answer the question. The first return getJSON(wikiUrl + person.name);
statement is part of the .map()
method. To understand it, it may be necessary to understand how the map method works. It seems to be 'constructing' an array profiles
, filling it up with each index person
. In other words, with each iteration, profiles[each iteration] or profiles[person] =
assigned the return value of getJSON(wikiUrl + person.name)
. Once the profiles array construction is complete (array size should equal json.people.length
), it is returned via return profiles;
One additional comment: I couldn't manage to see the profiles
array while it was 'in construction'. I tried with debugger and stepping in each iteration. I could only see contents of profiles
after it was fully built.
I did a simplified example here of the .map
method itself without the added complication of promise
concept.
let Arg = {
message: "Any message",
number: 4,
people: [
{
Name: "Jasmine",
Location: "Vienna"
},
{
Name: "Jason",
Location: "Venice"
},
{
Name: "Sarah",
Location: "Toronto"
},
{
Name: "Albert",
Location: "St. Louis"
},
]
};
function getProfiles(json) {
const profiles = json.people.map(index => {
// console.log(index.Name + ", from " + index.Location);
return index.Name + ", from " + index.Location;
});
return profiles;
}
console.log( `this function getProfiles accepts an argument and the returns the value of 'profiles' is as follows...`);
console.log( getProfiles(Arg) );
//output :
// [
// "Jasmine, from Vienna",
// "Jason, from Venice",
// "Sarah, from Toronto",
// "Albert, from St. Louis"
// ]

JASON LEE
17,352 PointsI got an email notification but I guess Gareth deleted his answer.
To clarify, function(x) => {x}
and function(x) => {return x;}
is NOT the same.
function(x) => x
and function(x) => {return x;}
IS the same.
I'm fairly confident of this, as I had to learn the hard way debugging code; it was a topic on Promises, so debugging took a good hour or so.

Gareth Moore
7,724 PointsHahaha Yeah I deleted my answer but actually I had a good point. It was still returning in either case but I had the wrong syntax. After I mucked around with it for a little while I got it to work. So I should have just edited my answer but I was like OMG I am a doofus, I should remove that hahaha
Indeed it was the curly brackets. If you have a single expression and no brackets then function(x) => x
will return
x.
In the video you can just change the function syntax and avoid using the return
keyword or you could put it in. Either way worked.