Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial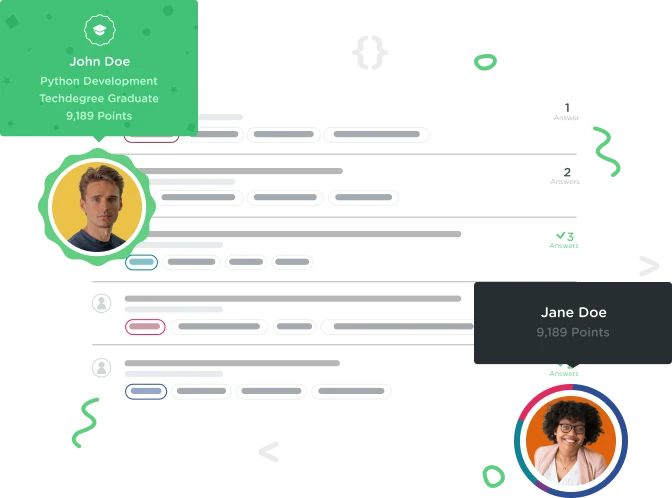

Susan Rusie
10,671 PointsWhat is going on with my application?
Whenever I type in a name, I get the name of the person and the edit and remove button, but I also get an empty editing box with the those same two buttons to the right of each name I type in. I have looked at the code and it looks right. What could be causing this to happen? Here is my code:
const form = document.getElementById('registrar');
const input = form.querySelector('input');
const ul = document.getElementById('invitedList');
function createLI(text) {
const li = document.createElement('li');
li.textContent = text;
const label = document.createElement('label');
label.textContent = 'Confirmed';
const checkbox = document.createElement('input');
checkbox.type = 'checkbox';
label.appendChild(checkbox);
li.appendChild(label);
const editButton = document.createElement('button');
editButton.textContent = 'edit';
li.appendChild(editButton);
const removeButton = document.createElement('button');
removeButton.textContent = 'remove';
li.appendChild(removeButton);
return li;
}
form.addEventListener('submit', (e) => {
e.preventDefault();
const text = input.value;
input.value = '';
const li = createLI(text);
ul.appendChild(li);
});
ul.addEventListener('change', (e) => {
const checkbox = event.target;
const checked = checkbox.checked;
const listItem = checkbox.parentNode.parentNode;
if (checked) {
listItem.className = 'responded';
} else {
listItem.className = '';
}
});
ul.addEventListener('click', (e) => {
if (e.target.tagName === 'BUTTON') {
const li = e.target.parentNode;
const ul = li.parentNode;
ul.removeChild(li);
}
});
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>RSVP App</title>
<link href="https://fonts.googleapis.com/css?family=Courgette" rel="stylesheet">
<link href="https://fonts.googleapis.com/css?family=Lato:400,700" rel="stylesheet">
<link href="css/style.css" rel="stylesheet">
</head>
<body>
<div class="wrapper">
<header>
<h1>RSVP</h1>
<p>A Treehouse App</p>
<form id="registrar">
<input type="text" name="name" placeholder="Invite Someone">
<button type="submit" name="submit" value="submit">Submit</button>
</form>
</header>
<div class="main">
<h2>Invitees</h2>
<ul id="invitedList"></ul>
</div>
</div>
<script type="text/javascript" src="app.js"></script>
</body>
</html>
2 Answers
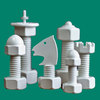
Steven Parker
231,269 PointsI don't see that behavior when I try the code.
But I did notice that all buttons act as "remove" buttons.
Perhaps there are contributing factors in other code parts not shown here. It might be a good idea to make a snapshot of your workspace and share the link to that.
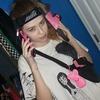
ohboy
2,232 PointsIf it looks like this http://prntscr.com/ivizra, then I would suggest re-opening your browser.
Susan Rusie
10,671 PointsSusan Rusie
10,671 PointsI am using my own text editor, so how would I do that?
Steven Parker
231,269 PointsSteven Parker
231,269 PointsYou could upload the files into a workspace, or you could post the rest of the source code here.
Susan Rusie
10,671 PointsSusan Rusie
10,671 PointsI posted the rest of my code. I tried it again and it doesn't always do that with all of the names. Sometimes, it is just one name if I select multiple names. I looked at my code again and still can't see what might be causing this problem. I have even checked the developer tools to see it showed any errors and there were none. If you have any ideas what may be causing this problem, I'm all ears. Any help you can offer is greatly appreciated.
Steven Parker
231,269 PointsSteven Parker
231,269 PointsCan you give me specific steps to reproduce the issue? I've tried creating up to 5 entries and removing them, but none had any extra buttons.
Susan Rusie
10,671 PointsSusan Rusie
10,671 PointsI would like to show you a screenshot of what I am seeing, but I don't know how to do that.
Steven Parker
231,269 PointsSteven Parker
231,269 PointsYou can't upload images. If they are hosted somewhere you can link them. But I was hoping you'd tell me what actions to take to create the problem (keystrokes, mouse clicks, etc).
Susan Rusie
10,671 PointsSusan Rusie
10,671 PointsUnfortunately, I am not doing anything different than what he is doing in the video. I even tested his "final" code for this video to see if I get the same results, which I did. It may be something screwy with the browser itself, but I was able to get through the video getting the same result he was with "edit" printing to the console when I clicked on the button. I don't know what is causing it. I guess I will just have to be satisfied that I got through the video and can move on to the next video in this course.
Steven Parker
231,269 PointsSteven Parker
231,269 PointsOK, but you might also consider the possibility of a bug in the editor that is simulating the browser for you.